web控制串口.html,[转]web串口调试助手,浏览器控制串口设备
时间: 2023-11-27 11:55:05 浏览: 108
很好,这是一个用于在浏览器中控制串口设备的Web应用程序。它可以帮助您进行串口调试和测试。该应用程序使用JavaScript编写,利用Web API与串口进行通信。
该应用程序具有以下功能:
1. 打开和关闭串口设备
2. 设置串口参数,如波特率、数据位、停止位和校验位
3. 发送数据到串口设备
4. 接收来自串口设备的数据,并在浏览器中显示
您可以将该应用程序部署到Web服务器上,或者直接从本地文件系统中运行。请注意,在实际使用中,您需要将应用程序修改为符合您的具体需求。
下面是该应用程序的代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Web Serial Terminal</title>
</head>
<body>
<h1>Web Serial Terminal</h1>
<form>
<fieldset>
<legend>Serial Port Settings</legend>
<label for="port-select">Select a serial port:</label>
<select id="port-select"></select>
<label for="baud-rate-select">Baud rate:</label>
<select id="baud-rate-select">
<option value="9600">9600</option>
<option value="19200">19200</option>
<option value="38400">38400</option>
<option value="57600">57600</option>
<option value="115200">115200</option>
</select>
<label for="data-bits-select">Data bits:</label>
<select id="data-bits-select">
<option value="8">8</option>
<option value="7">7</option>
<option value="6">6</option>
<option value="5">5</option>
</select>
<label for="stop-bits-select">Stop bits:</label>
<select id="stop-bits-select">
<option value="1">1</option>
<option value="2">2</option>
</select>
<label for="parity-select">Parity:</label>
<select id="parity-select">
<option value="none">None</option>
<option value="even">Even</option>
<option value="odd">Odd</option>
<option value="mark">Mark</option>
<option value="space">Space</option>
</select>
</fieldset>
<fieldset>
<legend>Send Data</legend>
<label for="send-textarea">Text to send:</label>
<textarea id="send-textarea" rows="4"></textarea>
<button type="button" id="send-button">Send</button>
</fieldset>
<fieldset>
<legend>Receive Data</legend>
<pre id="receive-pre"></pre>
</fieldset>
</form>
<script>
const portSelect = document.getElementById('port-select');
const baudRateSelect = document.getElementById('baud-rate-select');
const dataBitsSelect = document.getElementById('data-bits-select');
const stopBitsSelect = document.getElementById('stop-bits-select');
const paritySelect = document.getElementById('parity-select');
const sendTextarea = document.getElementById('send-textarea');
const sendButton = document.getElementById('send-button');
const receivePre = document.getElementById('receive-pre');
let port;
async function getPorts() {
const ports = await navigator.serial.getPorts();
return ports.map(port => port.path);
}
async function openPort(path, baudRate, dataBits, stopBits, parity) {
const serialOptions = {
baudRate: parseInt(baudRate),
dataBits: parseInt(dataBits),
stopBits: parseInt(stopBits),
parity: parity
};
port = await navigator.serial.requestPort({
filters: [{ usbVendorId: 0xXXXX, usbProductId: 0xXXXX }]
});
await port.open(serialOptions);
}
async function closePort() {
if (port) {
await port.close();
port = null;
}
}
async function sendText(text) {
if (port) {
const writer = port.writable.getWriter();
await writer.write(new TextEncoder().encode(text));
writer.releaseLock();
}
}
async function receiveText() {
if (port) {
const reader = port.readable.getReader();
try {
while (true) {
const { value, done } = await reader.read();
if (done) {
break;
}
receivePre.textContent += new TextDecoder().decode(value);
}
} catch (error) {
console.error(error);
} finally {
reader.releaseLock();
}
}
}
async function updatePorts() {
const ports = await getPorts();
portSelect.innerHTML = '';
ports.forEach(port => {
const option = document.createElement('option');
option.value = port;
option.textContent = port;
portSelect.appendChild(option);
});
}
async function onUpdatePortsButtonClick() {
await updatePorts();
}
async function onOpenPortButtonClick() {
const path = portSelect.value;
const baudRate = baudRateSelect.value;
const dataBits = dataBitsSelect.value;
const stopBits = stopBitsSelect.value;
const parity = paritySelect.value;
await openPort(path, baudRate, dataBits, stopBits, parity);
}
async function onClosePortButtonClick() {
await closePort();
}
async function onSendButtonClick() {
const text = sendTextarea.value;
await sendText(text);
}
async function onReceiveButtonClick() {
await receiveText();
}
async function init() {
const ports = await getPorts();
ports.forEach(port => {
const option = document.createElement('option');
option.value = port;
option.textContent = port;
portSelect.appendChild(option);
});
sendButton.addEventListener('click', onSendButtonClick);
}
init();
</script>
</body>
</html>
```
请注意,该应用程序依赖于Web Serial API,因此只能在支持该API的浏览器中运行。目前,Chrome和Edge是支持该API的浏览器。
阅读全文
相关推荐
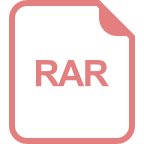
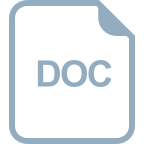

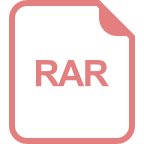
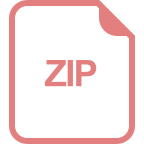
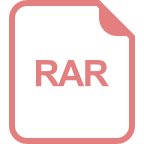
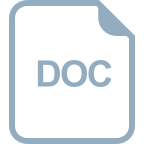
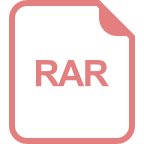
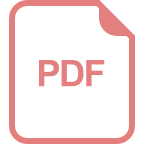
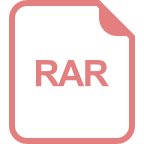
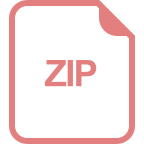
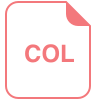
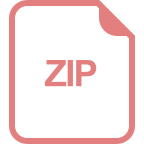
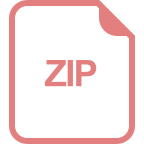
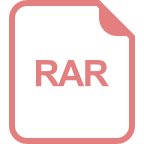