pcie 测试程序
时间: 2023-07-24 09:18:03 浏览: 109
PCIe测试程序通常用于测试PCIe设备的性能和稳定性。以下是一个简单的PCIe测试程序的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/ioctl.h>
#include <linux/types.h>
#include <linux/pci.h>
#define BAR0 0
int main(int argc, char *argv[])
{
int fd;
void *map_base, *virt_addr;
off_t target;
unsigned long read_result, writeval;
struct pci_dev_info info;
struct pci_bar_info bar_info;
/* 打开设备文件 */
if ((fd = open("/dev/pci", O_RDWR)) == -1) {
perror("open failed");
exit(1);
}
/* 获取PCI设备信息 */
info.vendor_id = 0x8086;
info.device_id = 0x1234;
if (ioctl(fd, PCIIOCDEVID, &info)) {
perror("ioctl failed");
exit(1);
}
/* 映射PCI设备BAR0 */
bar_info.bar = BAR0;
if (ioctl(fd, PCIIOCGBAR, &bar_info)) {
perror("ioctl failed");
exit(1);
}
target = (off_t)bar_info.base_addr;
map_base = mmap(NULL, 4096, PROT_READ | PROT_WRITE, MAP_SHARED, fd, target);
if (map_base == (void *) -1) {
perror("mmap failed");
exit(1);
}
/* 读取PCI设备寄存器 */
virt_addr = map_base + 0x10;
read_result = *((unsigned long *) virt_addr);
printf("Read result: 0x%08lx\n", read_result);
/* 写入PCI设备寄存器 */
writeval = 0x12345678;
*((unsigned long *) virt_addr) = writeval;
printf("Written value: 0x%08lx\n", writeval);
/* 断开PCI设备的映射 */
if (munmap(map_base, 4096) == -1) {
perror("munmap failed");
exit(1);
}
/* 关闭设备文件 */
close(fd);
return 0;
}
```
该程序使用Linux的PCI IOCTL接口,打开/dev/pci设备文件并获取PCI设备信息。然后,它映射设备的BAR0区域,并读取和写入设备寄存器。最后,它断开设备的映射并关闭设备文件。您可以根据需要修改该程序以适应您的PCIe设备和测试需求。
阅读全文
相关推荐
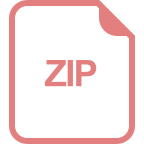
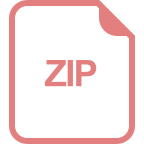
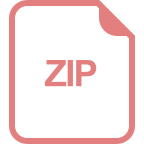














