如何通过AOP编程,对Repository层的方法进行拦截
时间: 2024-03-08 14:48:36 浏览: 162
可以通过在Spring中使用AOP编程,对Repository层的方法进行拦截,从而实现数据权限控制。具体步骤如下:
1. 定义一个切面类,实现`MethodInterceptor`接口,重写`invoke`方法,该方法将会在Repository层方法调用前后被执行。
```java
@Component
@Aspect
public class DataPermissionAspect implements MethodInterceptor {
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
// 在方法执行前处理数据权限控制逻辑
// ...
Object result = invocation.proceed();
// 在方法执行后处理数据权限控制逻辑
// ...
return result;
}
}
```
2. 在切面类上添加`@Aspect`注解,表明该类是一个切面类。
3. 使用`@Around`注解,将切面类的`invoke`方法与需要拦截的Repository层方法绑定起来。
```java
@Around("execution(* com.example.repository.*.*(..))")
public Object interceptRepositoryMethod(ProceedingJoinPoint joinPoint) throws Throwable {
// 在方法执行前处理数据权限控制逻辑
// ...
Object result = joinPoint.proceed();
// 在方法执行后处理数据权限控制逻辑
// ...
return result;
}
```
4. 在需要拦截的Repository层方法上添加自定义注解,表明该方法需要进行数据权限控制。
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
@DataPermission // 自定义注解
List<User> findByUsername(String username);
}
```
5. 在切面类中,根据自定义注解和当前用户的权限信息,动态修改SQL查询语句,实现数据权限控制。
```java
@Around("@annotation(DataPermission)")
public Object interceptRepositoryMethod(ProceedingJoinPoint joinPoint) throws Throwable {
// 获取当前用户的权限信息
// ...
// 获取需要拦截的Repository层方法的参数
Object[] args = joinPoint.getArgs();
// 动态修改SQL查询语句
String sql = "SELECT * FROM user WHERE 1=1";
if (hasPermission) {
sql += " AND user_id IN (SELECT user_id FROM user_role WHERE role_id = ?)";
args = ArrayUtils.add(args, roleId);
}
// 执行修改后的SQL查询语句
// ...
return result;
}
```
需要注意的是,通过AOP编程对Repository层方法进行拦截,需要深入了解AOP的原理和使用方法,同时也需要考虑性能和安全性等方面的问题。
阅读全文
相关推荐

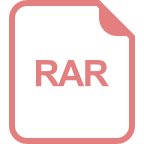



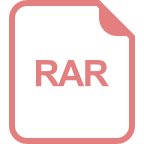











