C#遇到StackOverflowException报错如何通过代码解决
时间: 2023-12-10 16:42:12 浏览: 35
StackOverflowException 表示递归调用层数过多,超出了系统所能承受的最大深度,因此需要检查代码中是否存在无限递归的情况。
如果无法避免递归,可以通过以下方式增加堆栈大小来解决:
1. 在项目的属性设置中,选择“生成”选项卡,将“栈大小”设置为更大的值。
2. 在代码中使用尾递归,避免创建新的堆栈帧。
3. 优化递归算法,减少递归深度。
4. 将递归转换为循环或迭代实现。
以下是一个使用尾递归的示例:
```
public int Factorial(int n, int result = 1)
{
if (n == 0)
{
return result;
}
else
{
return Factorial(n - 1, result * n);
}
}
```
以上代码中,使用了尾递归来计算阶乘,避免了创建新的堆栈帧,从而减少了堆栈溢出的风险。
相关问题
c#:防止/处理StackOverflowException的代码
StackOverflowException是一种非常严重的异常,通常会导致应用程序崩溃。为了防止这种异常的发生,我们需要确保我们的递归调用具有结束条件,以便递归不会无限循环下去。
以下是一些防止/处理StackOverflowException的代码示例:
1. 增加递归深度限制
可以增加递归深度的限制,当递归深度超过一定限制时,停止递归。例如:
```
private const int MaxRecursionDepth = 1000;
private int recursionDepth = 0;
public void RecursiveMethod()
{
if (recursionDepth++ > MaxRecursionDepth)
{
throw new StackOverflowException("Recursive method exceeded maximum recursion depth.");
}
// Do recursive work here...
recursionDepth--;
}
```
2. 将递归转换为循环
可以将递归方法转换为循环方法,这样就避免了StackOverflowException。例如:
```
public void RecursiveMethod()
{
// Do some initial setup work here...
while (true)
{
// Do recursive work here...
if (/* some condition indicating we are done */)
{
break;
}
// Do some other work here...
}
// Do some final cleanup work here...
}
```
3. 使用尾递归
在某些编程语言中,可以使用尾递归来避免StackOverflowException。尾递归是指递归函数的最后一个操作是调用自身。这使得编译器可以优化递归,使其不会占用函数调用堆栈空间。例如:
```
public void RecursiveMethod(int n, int result)
{
if (n == 0)
{
Console.WriteLine(result);
return;
}
RecursiveMethod(n - 1, n * result);
}
```
C#Process is terminated due to StackOverflowException.
"C# Process is terminated due to StackOverflowException."是指在C#编程中,当执行代码时出现了StackOverflowException异常,即堆栈溢出异常。这个异常通常发生在无限递归调用时,即一个方法或属性无限地调用自身。根据引用所提供的代码示例,当对象为空时,使用"=="操作符进行比较会导致无限递归调用,最终导致堆栈溢出异常。为了解决这个问题,可以通过调用基类的操作符来打破无休止的递归调用。在给定的示例中,重载了"=="和"!="操作符来处理比较操作,通过引用基类object的比较操作符来避免无限递归调用的问题。这样可以避免出现StackOverflowException异常。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [C#重载操作符“==”执行时引发“Process is terminated due to StackOverflowException.”的解决办法](https://blog.csdn.net/wonsoft/article/details/6610245)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [libata-transport.rar_Terminated](https://download.csdn.net/download/weixin_42665255/86613946)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [C#程序出现Process is terminated due to StackOverflowException.---属性和字段混淆!](https://blog.csdn.net/aruewds/article/details/109324420)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
相关推荐
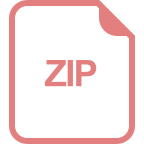












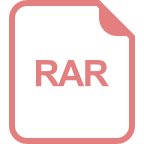