SPring IOC构造注入,生成一个eclipse程序
时间: 2024-09-15 07:04:59 浏览: 58
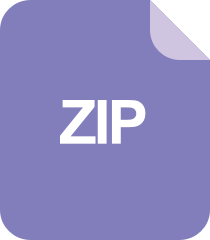
Spring_0300_IOC_Injection_Type 构造器注入
Spring框架的控制反转(IoC,Inversion of Control)是一种设计原则,用于减少代码之间的耦合度。通过这种方式,对象的创建和依赖关系的维护转移到了容器手中,从而实现了松耦合。构造注入是IoC的一种实现方式,它通过构造函数来注入依赖项。
以下是一个简单的例子,演示如何使用构造注入的方式在Spring框架中生成一个Eclipse环境下的程序:
首先,你需要在你的项目中添加Spring框架的依赖,如果你使用的是Maven,可以在`pom.xml`中添加如下依赖:
```xml
<dependencies>
<!-- 添加Spring的核心库 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.18</version>
</dependency>
<!-- 其他依赖 -->
</dependencies>
```
然后,创建一个服务类和一个客户端类,服务类通过构造函数注入:
```java
// Service类,定义了业务逻辑
public class MyService {
private String name;
public MyService(String name) {
this.name = name;
}
public void doSomething() {
System.out.println("Service doSomething by " + name);
}
}
// Client类,使用Service类
public class MyClient {
private MyService myService;
public MyClient(MyService myService) {
this.myService = myService;
}
public void useService() {
myService.doSomething();
}
}
```
接下来,配置Spring的applicationContext.xml文件,以使用构造注入的方式来装配这些类:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 配置Service -->
<bean id="myService" class="com.example.MyService">
<constructor-arg value="Spring"/>
</bean>
<!-- 配置Client,注入Service -->
<bean id="myClient" class="com.example.MyClient">
<constructor-arg ref="myService"/>
</bean>
</beans>
```
最后,创建一个主程序类来启动Spring容器,并获取Client的bean来运行程序:
```java
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class App {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
MyClient myClient = (MyClient) context.getBean("myClient");
myClient.useService();
}
}
```
以上步骤展示了如何在Spring中通过构造注入的方式创建对象和依赖关系,并在Eclipse环境中运行一个简单的Spring程序。
阅读全文
相关推荐
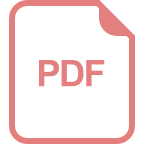
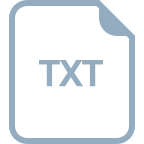
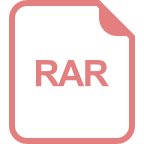
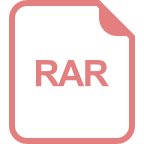
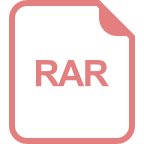
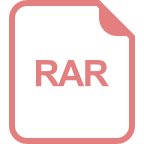
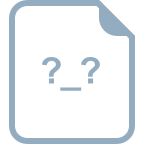
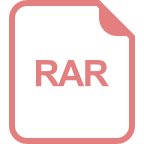
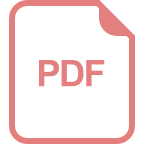
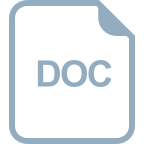
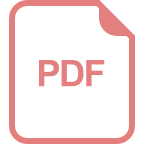
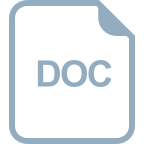
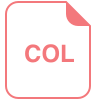
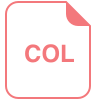
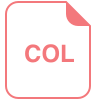
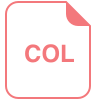
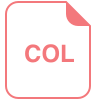
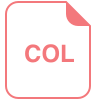