tkinter内嵌动态变化曲线代码
时间: 2023-07-09 11:35:59 浏览: 206
以下是一个简单的 tkinter 程序,它使用 matplotlib 库来绘制动态变化的曲线:
```python
import tkinter as tk
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
from matplotlib.figure import Figure
import numpy as np
class PlotWindow(tk.Frame):
def __init__(self, master=None):
tk.Frame.__init__(self, master)
self.create_widgets()
def create_widgets(self):
self.fig = Figure(figsize=(6, 4), dpi=100)
self.ax = self.fig.add_subplot(111)
self.ax.set_ylim(-1, 1)
self.canvas = FigureCanvasTkAgg(self.fig, self)
self.canvas.get_tk_widget().pack(side=tk.TOP, fill=tk.BOTH, expand=1)
self.button = tk.Button(self, text="Start", command=self.start_animation)
self.button.pack(side=tk.BOTTOM)
def start_animation(self):
self.x = np.linspace(0, 10, 100)
self.y = np.sin(self.x)
self.line, = self.ax.plot(self.x, self.y)
self.animation_id = self.after(100, self.update_animation)
def update_animation(self):
self.x += 0.1
self.y = np.sin(self.x)
self.line.set_data(self.x, self.y)
self.ax.relim()
self.ax.autoscale_view(True, True, True)
self.canvas.draw()
self.animation_id = self.after(100, self.update_animation)
if __name__ == "__main__":
root = tk.Tk()
plot_window = PlotWindow(root)
plot_window.pack(side=tk.TOP, fill=tk.BOTH, expand=1)
root.mainloop()
```
在这个程序中,我们首先创建了一个窗口,并在窗口中添加一个 matplotlib 图形,并添加一个“Start”按钮。当用户点击按钮时,我们会开始绘制一个正弦曲线,并使用 `after` 函数来更新曲线的动画效果。
当用户点击“Start”按钮时,我们会调用 `start_animation` 函数。在该函数中,我们首先定义了 `x` 和 `y` 数组,它们分别表示曲线的 x 和 y 值。然后我们使用 `ax.plot` 函数来绘制曲线,并将返回值保存在 `line` 变量中。最后,我们使用 `after` 函数来调用 `update_animation` 函数,以便每隔 100 毫秒更新一次曲线。
在 `update_animation` 函数中,我们首先更新 `x` 和 `y` 数组,然后使用 `line.set_data` 函数来更新曲线的数据。接着,我们使用 `ax.relim` 和 `ax.autoscale_view` 函数来调整曲线的缩放比例。最后,我们使用 `canvas.draw` 函数来更新图形的显示。
阅读全文
相关推荐
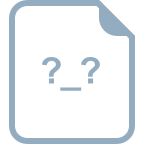
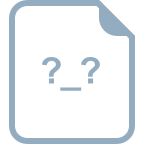
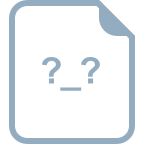
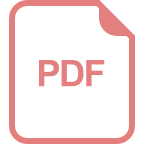
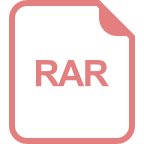
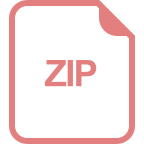
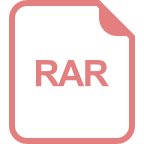
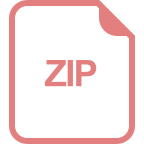
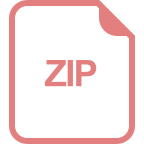
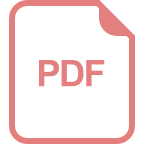
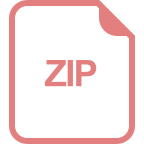
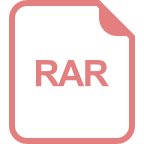
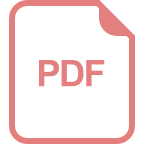
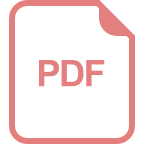
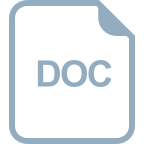
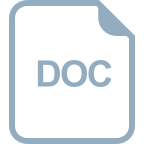
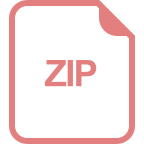