倒排索引并检索 java
时间: 2023-10-16 22:21:25 浏览: 98
倒排索引是一种数据结构,用于快速查找包含特定单词的文档或记录。在倒排索引中,每个单词都映射到包含该单词的文档或记录的列表,这些列表称为倒排列表。倒排索引常用于搜索引擎中,可以快速地找到包含用户查询关键字的文档。
在Java中,可以使用HashMap和HashSet来实现倒排索引。首先需要将文档分词,将每个单词作为键,对应的文档编号或文档对象作为值,存储到HashMap中。如果一个单词在多个文档中出现,需要将文档编号或文档对象添加到对应的倒排列表中。
检索时,将查询关键字作为键,从HashMap中获取对应的倒排列表,然后遍历列表,获取对应的文档编号或文档对象,即可得到搜索结果。
下面是一个简单的Java代码示例:
```
import java.util.*;
public class InvertedIndex {
private Map<String, Set<Integer>> index;
public InvertedIndex() {
index = new HashMap<>();
}
public void addDocument(int docId, String[] words) {
for (String word : words) {
if (!index.containsKey(word)) {
index.put(word, new HashSet<Integer>());
}
index.get(word).add(docId);
}
}
public Set<Integer> search(String query) {
return index.get(query);
}
public static void main(String[] args) {
InvertedIndex index = new InvertedIndex();
index.addDocument(1, new String[]{"apple", "banana", "orange"});
index.addDocument(2, new String[]{"apple", "pear", "peach"});
index.addDocument(3, new String[]{"banana", "pear", "grape"});
Set<Integer> result = index.search("apple");
System.out.println(result); // output: [1, 2]
}
}
```
阅读全文
相关推荐
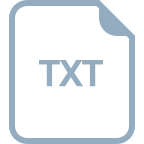
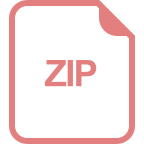
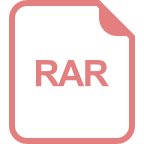
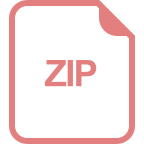
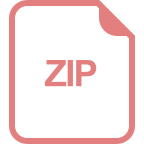
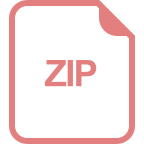
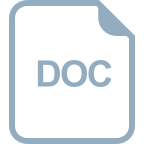
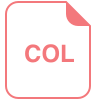
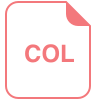
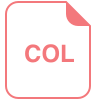
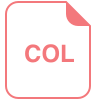


