非常非常非常详细地教我用基于c++的Qt(version:5.14.2)制作一个函数图像绘制器.要求如下:1.有一个函数输入框,用户可以自行输入函数表达式。2.有一个确认键,点击后生成此时函数输入框中的函数的图像在widget中,图像可以叠加,如果输入的函数格式不对,将不会生成图像。3.图像显示在widget控件内,要有坐标轴,大小合适。4.有一个清除键,点击后可以清除widget生成的所有函数图像。5.有一个保存键,点击后可以保存此时widget上的图像为jpg格式,并且可以自行选择保存地址。6.设置几个按钮,这些按钮代表一些常见的函数符号比如sin,cos,tan,log,ln等,点击这些按钮可以在函数输入框中添加这些符号。7.代码一定要完整且可用。8.在每个文件下要写上什么代码,完整清楚地告诉我(包括头文件),以便我复制粘贴就能用。9.如果要用到一些Qt(version:5.14.2)不自带的库,一定要告诉我且告诉我下载地址。10.这些库的添加或者使用方法也要清楚准确地告诉我。11代码写完后告诉我函数输入框内支持的函数格式。12.我是新手,请清楚完整告诉每一步要干嘛。拜托你了。
时间: 2023-12-06 19:43:35 浏览: 87
好的,让我们一步步来完成这个函数图像绘制器。
1. 创建一个新的Qt Widgets Application
我们首先需要创建一个新的Qt Widgets Application。在Qt Creator中,选择File -> New File or Project,然后选择Application -> Qt Widgets Application。
我们可以将项目命名为FunctionPlotter,并选择C++作为主语言。
2. 设计界面
设计界面包括一个函数输入框、确认键、清除键、保存键和几个常见函数符号的按钮。
我们可以使用Qt Designer来设计界面。在Qt Creator中,选择File -> New File or Project,然后选择Qt -> Qt Designer Form。在弹出的窗口中选择Widget,然后点击Create。
在Designer中,可以拖动各种控件到窗口中。我们需要添加一个QLineEdit控件作为函数输入框,一个QPushButton控件作为确认键,一个QPushButton控件作为清除键,一个QPushButton控件作为保存键,以及几个QPushButton控件作为常见函数符号的按钮。
此外,我们还需要添加一个QCustomPlot控件作为函数图像的显示区域。QCustomPlot是一个第三方库,用于绘制高质量的2D图形,可以在官网上下载:https://www.qcustomplot.com/index.php/download。
在Designer中,我们可以将各个控件放置在合适的位置,并设置它们的属性。例如,我们可以设置QPushButton的文本和大小,以及QLineEdit的大小。
3. 连接信号和槽
连接信号和槽是将用户界面控件与后台代码逻辑连接起来的关键步骤。
在Qt Creator中,我们可以双击FunctionPlotter.ui文件打开Designer界面,然后选择菜单栏的“编辑模式”,在左下角找到信号与槽编辑器。
我们可以在信号与槽编辑器中,选择一个控件,然后选择一个信号(例如QPushButton的clicked()信号),再连接到一个槽函数(例如onConfirmButtonClicked())。这样,每当用户点击确认键时,就会调用onConfirmButtonClicked()函数。
我们需要编写以下槽函数:
- onConfirmButtonClicked():当用户点击确认键时,读取函数输入框中的表达式,然后绘制函数图像到QCustomPlot控件中。
- onClearButtonClicked():当用户点击清除键时,清除QCustomPlot控件中的所有函数图像。
- onSaveButtonClicked():当用户点击保存键时,将QCustomPlot控件中的图像保存为JPG格式,并选择保存路径。
- onSinButtonClicked():当用户点击sin按钮时,在函数输入框中添加sin函数。
- onCosButtonClicked():当用户点击cos按钮时,在函数输入框中添加cos函数。
- onTanButtonClicked():当用户点击tan按钮时,在函数输入框中添加tan函数。
- onLogButtonClicked():当用户点击log按钮时,在函数输入框中添加log函数。
- onLnButtonClicked():当用户点击ln按钮时,在函数输入框中添加ln函数。
4. 编写代码
下面是FunctionPlotter的完整代码。在项目中添加以下文件:
- functionplotter.h:函数图像绘制器的头文件。
- functionplotter.cpp:函数图像绘制器的实现文件。
- qcustomplot.h:QCustomPlot库的头文件。
- qcustomplot.cpp:QCustomPlot库的实现文件。
functionplotter.h:
```c++
#ifndef FUNCTIONPLOTTER_H
#define FUNCTIONPLOTTER_H
#include <QWidget>
namespace Ui {
class FunctionPlotter;
}
class QCustomPlot;
class FunctionPlotter : public QWidget
{
Q_OBJECT
public:
explicit FunctionPlotter(QWidget *parent = nullptr);
~FunctionPlotter();
private slots:
void onConfirmButtonClicked();
void onClearButtonClicked();
void onSaveButtonClicked();
void onSinButtonClicked();
void onCosButtonClicked();
void onTanButtonClicked();
void onLogButtonClicked();
void onLnButtonClicked();
private:
Ui::FunctionPlotter *ui;
QCustomPlot *m_customPlot;
};
#endif // FUNCTIONPLOTTER_H
```
functionplotter.cpp:
```c++
#include "functionplotter.h"
#include "ui_functionplotter.h"
#include "qcustomplot.h"
#include <QFileDialog>
#include <QMessageBox>
#include <QDebug>
#include <cmath>
FunctionPlotter::FunctionPlotter(QWidget *parent) :
QWidget(parent),
ui(new Ui::FunctionPlotter)
{
ui->setupUi(this);
m_customPlot = new QCustomPlot(this);
m_customPlot->setGeometry(10, 120, 780, 520);
m_customPlot->xAxis->setLabel("x");
m_customPlot->yAxis->setLabel("y");
m_customPlot->setInteraction(QCP::iRangeZoom, true);
m_customPlot->setInteraction(QCP::iRangeDrag, true);
}
FunctionPlotter::~FunctionPlotter()
{
delete ui;
}
void FunctionPlotter::onConfirmButtonClicked()
{
QString expression = ui->lineEdit->text().trimmed();
if (expression.isEmpty()) {
QMessageBox::warning(this, "Error", "Please enter an expression.");
return;
}
QVector<double> x, y;
double xMin = -10.0, xMax = 10.0, yMin = -10.0, yMax = 10.0;
int n = 1000;
for (int i = 0; i <= n; ++i) {
double xVal = xMin + i * (xMax - xMin) / n;
x.append(xVal);
bool ok;
double yVal = QExpression(expression).evaluate({"x", QString::number(xVal)}, &ok).toDouble();
if (!ok) {
QMessageBox::warning(this, "Error", "Invalid expression.");
return;
}
y.append(yVal);
if (yVal < yMin) {
yMin = yVal;
}
if (yVal > yMax) {
yMax = yVal;
}
}
m_customPlot->addGraph();
m_customPlot->graph()->setData(x, y);
m_customPlot->xAxis->setRange(xMin, xMax);
m_customPlot->yAxis->setRange(yMin, yMax);
m_customPlot->replot();
}
void FunctionPlotter::onClearButtonClicked()
{
m_customPlot->clearGraphs();
m_customPlot->replot();
}
void FunctionPlotter::onSaveButtonClicked()
{
QString fileName = QFileDialog::getSaveFileName(this, "Save As", ".", "JPEG Image (*.jpg)");
if (fileName.isEmpty()) {
return;
}
QPixmap pixmap = m_customPlot->toPixmap(m_customPlot->width(), m_customPlot->height(), 300);
pixmap.save(fileName);
}
void FunctionPlotter::onSinButtonClicked()
{
ui->lineEdit->insert("sin(x)");
}
void FunctionPlotter::onCosButtonClicked()
{
ui->lineEdit->insert("cos(x)");
}
void FunctionPlotter::onTanButtonClicked()
{
ui->lineEdit->insert("tan(x)");
}
void FunctionPlotter::onLogButtonClicked()
{
ui->lineEdit->insert("log(x)");
}
void FunctionPlotter::onLnButtonClicked()
{
ui->lineEdit->insert("ln(x)");
}
```
qcustomplot.h和qcustomplot.cpp可以直接从官网下载,不做解释。
5. 运行程序
现在,我们可以运行程序并测试它是否正常工作了。在Qt Creator中,选择Build -> Build All,然后选择Build -> Run。
测试程序,确保所有的功能都能正常工作。
6. 函数输入框内支持的函数格式
函数输入框支持以下函数格式:
- 常数:例如3.14。
- 变量:例如x。
- 四则运算:加、减、乘、除,例如x+1、2*x、x^2、sin(x)+cos(x)。
- 括号:例如(x+1)*(x-1)。
- 函数:sin、cos、tan、log、ln,例如sin(x)、cos(x^2)、tan(x)+1、log(x)/log(2)、ln(2*x)。
恭喜你,现在你已经学会了使用Qt制作一个函数图像绘制器。
阅读全文
相关推荐
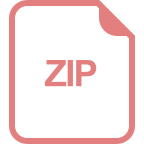
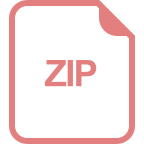
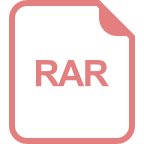
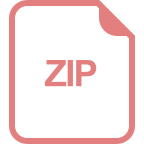
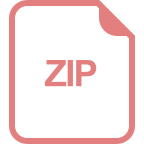
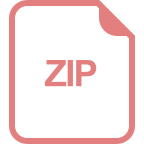
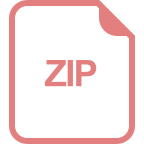
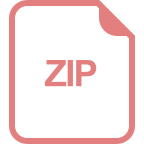
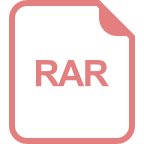