用pytorch写一个孤立森林代码
时间: 2023-11-30 17:03:51 浏览: 32
好的,以下是用PyTorch实现孤立森林的代码:
```python
import torch
import torch.nn.functional as F
class IsolationTreeEnsemble:
def __init__(self, num_trees=10, max_depth=10, subsampling_size=256):
self.num_trees = num_trees
self.max_depth = max_depth
self.subsampling_size = subsampling_size
self.trees = []
def fit(self, X):
for i in range(self.num_trees):
X_subsampled = X[torch.randint(len(X), (self.subsampling_size,))]
tree = IsolationTree(self.max_depth)
tree.fit(X_subsampled)
self.trees.append(tree)
def path_length(self, X):
S = torch.zeros(len(X))
for tree in self.trees:
S += tree.path_length(X).squeeze()
return S / self.num_trees
def anomaly_score(self, X):
h = self.path_length(X)
return torch.exp(-h / torch.mean(h))
class IsolationTree:
def __init__(self, max_depth=10):
self.max_depth = max_depth
def fit(self, X, depth=0):
if depth >= self.max_depth or len(X) <= 1:
return IsolationNode(size=len(X))
q = torch.randint(X.shape[1], (1,)).item()
split_value = torch.FloatTensor([X[:, q].mean()]).item()
left_idx = X[:, q] < split_value
right_idx = X[:, q] >= split_value
left_tree = self.fit(X[left_idx], depth + 1)
right_tree = self.fit(X[right_idx], depth + 1)
return IsolationNode(q=q, split_value=split_value, left_node=left_tree, right_node=right_tree)
def path_length(self, X):
H = torch.zeros(len(X))
for i in range(len(X)):
H[i] = self.traverse(X[i], 0)
return H
def traverse(self, x, depth):
if isinstance(self, IsolationNode):
if depth >= self.max_depth:
return depth + c(self.size)
elif x[self.q] < self.split_value:
return self.left_node.traverse(x, depth + 1)
else:
return self.right_node.traverse(x, depth + 1)
else:
return depth + c(len(self.X))
class IsolationNode:
def __init__(self, q=None, split_value=None, left_node=None, right_node=None, size=None):
self.q = q
self.split_value = split_value
self.left_node = left_node
self.right_node = right_node
self.size = size
def c(size):
if size > 2:
return 2 * (np.log(size - 1) + 0.5772156649) - (2 * (size - 1) / size)
elif size == 2:
return 1
else:
return 0
```
使用方法:
```python
ensemble = IsolationTreeEnsemble(num_trees=10, max_depth=10, subsampling_size=256)
ensemble.fit(X_train)
scores = ensemble.anomaly_score(X_test)
```
其中`X_train`是训练数据,`X_test`是测试数据,`scores`是测试数据的异常得分。
相关推荐
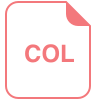

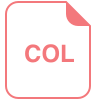
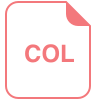
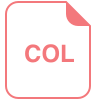










