python twisted autobahn
时间: 2023-09-26 09:12:21 浏览: 49
Twisted 是 Python 语言中的一个异步网络编程框架,而 Autobahn 是基于 Twisted 的 WebSocket 库和 WAMP(Web Application Messaging Protocol)实现。Autobahn 可以在 Twisted 环境下使用,并且 Autobahn 提供了 Twisted 集成的功能。具体来说,Autobahn 提供了 Twisted 的 Protocol 和 Transport 的实现,同时还提供了用于处理 WebSocket 和 WAMP 的 Twisted 协议工厂。
使用 Autobahn 需要先安装 Twisted,然后安装 Autobahn。安装完成后,可以使用 Autobahn 提供的 WebSocketServerProtocol 和 WebSocketServerFactory 类来实现 WebSocket 服务器,并使用 Autobahn 提供的 WebSocketClientProtocol 和 WebSocketClientFactory 类来实现 WebSocket 客户端。同时,Autobahn 还提供了 WampServerProtocol 和 WampServerFactory 类来实现 WAMP 服务器,以及 WampClientProtocol 和 WampClientFactory 类来实现 WAMP 客户端。
总之,Twisted 和 Autobahn 结合使用可以实现高效的异步 WebSocket 和 WAMP 服务器和客户端,适用于实时通信等场景。
相关问题
python twisted autobahn程序举例
下面是一个使用 Twisted 和 Autobahn 实现的简单 WebSocket 服务器程序的示例:
```python
from twisted.internet import reactor
from autobahn.twisted.websocket import WebSocketServerFactory, WebSocketServerProtocol
class MyServerProtocol(WebSocketServerProtocol):
def onOpen(self):
print("WebSocket connection opened")
def onMessage(self, payload, isBinary):
if isBinary:
print("Binary message received: {} bytes".format(len(payload)))
else:
print("Text message received: {}".format(payload.decode('utf8')))
if __name__ == '__main__':
factory = WebSocketServerFactory()
factory.protocol = MyServerProtocol
reactor.listenTCP(9000, factory)
print("WebSocket server listening on port 9000")
reactor.run()
```
这个程序创建了一个 WebSocket 服务器,监听本地的 9000 端口,并通过 Autobahn 提供的 WebSocketServerProtocol 类来实现 WebSocket 协议。当有客户端连接到服务器时,会调用 onOpen 方法;当服务器收到客户端发送的消息时,会调用 onMessage 方法进行处理。在这个示例中,只是简单地输出收到的消息。最后使用 Twisted 的 reactor 来运行服务器。
这个示例只是一个简单的 WebSocket 服务器程序,Autobahn 还提供了更多功能,例如支持 SSL 加密、支持 WAMP 协议等。
python twisted autobahn发送消息
在 Autobahn 中,WebSocket 客户端和服务器端都可以通过 send 方法来发送消息,具体方法如下:
```python
class MyServerProtocol(WebSocketServerProtocol):
def onMessage(self, payload, isBinary):
if isBinary:
print("Binary message received: {} bytes".format(len(payload)))
else:
print("Text message received: {}".format(payload.decode('utf8')))
# 发送消息
self.sendMessage(payload, isBinary=isBinary)
```
在这个示例中,当服务器收到客户端发送的消息时,会通过 sendMessage 方法将消息发送回客户端。sendMessage 方法的第一个参数是消息的内容,第二个参数指示消息是否是二进制消息。
类似地,WebSocket 客户端也可以通过 send 方法来发送消息,具体方法如下:
```python
from twisted.internet import reactor
from autobahn.twisted.websocket import WebSocketClientFactory, WebSocketClientProtocol
class MyClientProtocol(WebSocketClientProtocol):
def onOpen(self):
print("WebSocket connection opened")
# 发送消息
self.sendMessage("Hello, world!".encode('utf8'))
def onMessage(self, payload, isBinary):
if isBinary:
print("Binary message received: {} bytes".format(len(payload)))
else:
print("Text message received: {}".format(payload.decode('utf8')))
if __name__ == '__main__':
factory = WebSocketClientFactory()
factory.protocol = MyClientProtocol
reactor.connectTCP("localhost", 9000, factory)
print("WebSocket client connected to server")
reactor.run()
```
在这个示例中,当客户端连接到服务器时,会调用 onOpen 方法,在这个方法中可以发送消息。在这个示例中,发送了一个文本消息。在 onMessage 方法中,处理服务器发送的消息,这里只是简单地输出收到的消息。
相关推荐
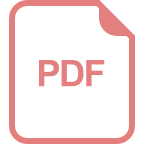
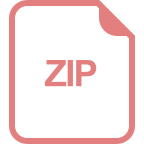












