golang 动态装载 protobuf 格式
时间: 2023-09-02 21:07:29 浏览: 244
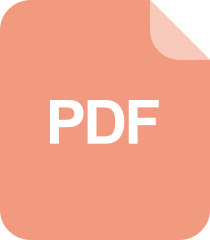
golang 微服务之gRPC与Protobuf的使用
在 Go 语言中,可以使用 `proto.Unmarshal` 函数将 protobuf 格式的数据反序列化为结构体对象。如果要动态装载 protobuf 格式的数据,可以先使用 `proto.Unmarshal` 函数将数据反序列化为 `proto.Message` 对象,然后使用反射(reflection)机制获取该对象的类型信息,并创建一个新的结构体对象来装载数据。
以下是一个示例代码,演示了如何动态装载 protobuf 格式的数据:
```go
package main
import (
"fmt"
"reflect"
"github.com/golang/protobuf/proto"
)
type Person struct {
Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"`
Age int `protobuf:"varint,2,opt,name=age,proto3" json:"age,omitempty"`
}
func main() {
data := []byte{0x0a, 0x04, 0x4a, 0x6f, 0x68, 0x6e, 0x10, 0x1e}
p := &Person{}
msg := proto.MessageV2(p)
err := proto.Unmarshal(data, msg)
if err != nil {
fmt.Println(err)
}
fmt.Println(p)
// 动态装载数据
msgType := reflect.TypeOf(msg).Elem()
newP := reflect.New(msgType).Interface()
err = proto.Unmarshal(data, newP.(proto.Message))
if err != nil {
fmt.Println(err)
}
person := newP.(*Person)
fmt.Println(person)
}
```
在这个示例中,我们首先使用 `proto.Unmarshal` 函数将 protobuf 格式的数据反序列化为 `Person` 结构体对象,并打印出了该对象的值。然后,我们使用反射机制获取 `msg` 对象的类型信息,并创建一个新的结构体对象 `newP` 来装载数据。最后,我们将 `newP` 转换为 `*Person` 类型,并打印出了该对象的值。
需要注意的是,在使用反射机制进行动态装载时,应该根据具体的需求和场景考虑是否使用反射,以及如何进行反射操作。反射机制可能会带来一定的性能开销,并且可能会导致代码的可读性降低。
阅读全文
相关推荐
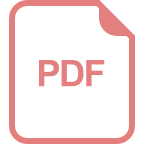
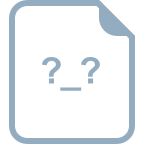















