9.建立一个汽车Auto类,包括轮胎个数、汽车颜色、车身重量、速度等成贝文里,开通过不同的构造方法创建实例。至少要求:汽车能够加速、减速、停车。再定义一个小汽车类Car,继承Auto,并添加空调、CD等成员变量,覆盖加
时间: 2024-12-18 08:26:24 浏览: 15
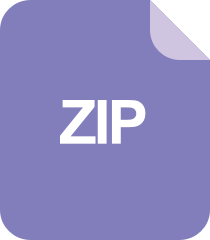
beven:旅行费用计算器和账单拆分
在面向对象编程中,你可以创建一个名为`Auto`的基础类,代表一般的汽车属性和行为,然后定义一个特殊类型的汽车`Car`作为其子类,增加额外的功能。这是一个基本的设计:
```java
// Auto类
public class Auto {
private int tireCount;
private String color;
private double bodyWeight;
private double speed;
// 构造方法
public Auto(int tireCount, String color, double bodyWeight) {
this.tireCount = tireCount;
this.color = color;
this.bodyWeight = bodyWeight;
this.speed = 0; // 初始化速度为0
}
// 普通方法
public void accelerate() { speed += 5; }
public void decelerate() { if (speed > 0) speed -= 5; }
public void stop() { speed = 0; }
// getter和setter方法
// ...
// 用于演示小汽车特有的方法
public void toggleAC() {
// 实现为空,小汽车类需要覆盖此方法
System.out.println("Air conditioning is disabled in the base Auto class");
}
}
// Car类,继承自Auto
public class Car extends Auto {
private boolean hasAC;
private boolean hasCD;
// 继承的构造方法
public Car(int tireCount, String color, double bodyWeight, boolean hasAC, boolean hasCD) {
super(tireCount, color, bodyWeight);
this.hasAC = hasAC;
this.hasCD = hasCD;
}
@Override
public void toggleAC() {
if (hasAC) {
System.out.println("Turning on air conditioner");
} else {
System.out.println("Air conditioner already off");
}
}
// 可能覆盖的其他方法,如toggleCD等
// 其他getter和setter方法
// ...
}
```
在这个例子中,`Car`类继承了`Auto`的共性,并覆盖了`toggleAC()`方法来体现小汽车的独特功能。你可以通过创建`Car`对象并调用它的方法来操作车辆,例如:
```java
Car myCar = new Car(4, "Red", 2000, true, true);
myCar.accelerate();
myCar.toggleAC(); // 调用小汽车特有的方法
```
阅读全文
相关推荐
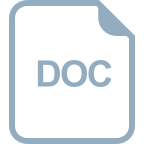
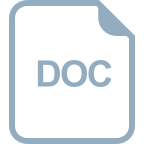
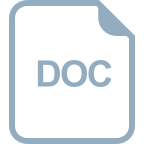
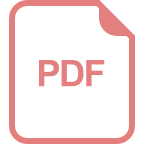
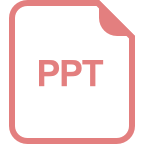
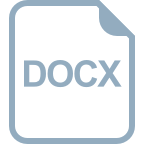
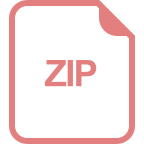