tc264串口通信接收数据
时间: 2023-07-25 11:03:48 浏览: 350
TC264是基于ARM Cortex-M4内核的微控制器,其串口通信接收数据的实现方式与其他微控制器类似。下面是一个简单的串口接收数据的示例程序:
```c
#include <stdio.h>
#include <stdint.h>
#include <stdbool.h>
#include "TC264.h"
#define UART_BAUDRATE 115200
#define UART_RX_BUFFER_SIZE 256
volatile uint8_t uart_rx_buffer[UART_RX_BUFFER_SIZE];
volatile uint8_t uart_rx_head = 0;
volatile uint8_t uart_rx_tail = 0;
void uart_init(void)
{
uint32_t baudrate_div = (uint32_t)((float)SystemCoreClock / (float)(16 * UART_BAUDRATE));
SCU_RESET->PRCLR0 |= SCU_RESET_PRCLR0_PERI_RESET_Msk; // reset UART peripheral
SCU_CLK->CLKCR |= SCU_CLK_CLKCR_UART_DIV_Msk; // enable UART clock
UART0->CR = 0; // disable UART
UART0->CR = UART_CR_RXEN_Msk | UART_CR_TXEN_Msk; // enable RX and TX
UART0->DIV = baudrate_div; // set baudrate
NVIC_EnableIRQ(UART0_IRQn); // enable UART interrupt
}
void UART0_IRQHandler(void)
{
uint8_t ch = UART0->RB;
uint8_t next_head = (uart_rx_head + 1) % UART_RX_BUFFER_SIZE;
if (next_head != uart_rx_tail) {
uart_rx_buffer[uart_rx_head] = ch;
uart_rx_head = next_head;
}
}
bool uart_read_byte(uint8_t *ch)
{
if (uart_rx_head == uart_rx_tail) {
return false;
}
*ch = uart_rx_buffer[uart_rx_tail];
uart_rx_tail = (uart_rx_tail + 1) % UART_RX_BUFFER_SIZE;
return true;
}
int main(void)
{
uart_init();
while (1) {
uint8_t ch;
if (uart_read_byte(&ch)) {
printf("Received: %c\n", ch);
}
}
return 0;
}
```
该程序初始化了UART0串口,使其以115200波特率接收数据。当有数据到达时,UART0_IRQHandler()函数会被调用,将接收到的字节存储到一个循环缓冲区中。程序的主循环中通过uart_read_byte()函数从缓冲区中读取一个字节,如果缓冲区为空则返回false。可以根据实际需要修改缓冲区大小、波特率等参数。
阅读全文
相关推荐






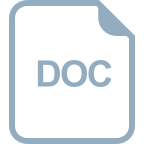








