c语言支持正则表达式查找
时间: 2024-10-19 17:14:18 浏览: 28
C语言本身并不直接内置对正则表达式的支持,因为标准库中没有提供相关的函数库。不过,有一些第三方库可以帮助你在C语言中使用正则表达式,如PCRE(Perl Compatible Regular Expressions)库或者Gnu regex库(即libregex或GLib)。
如果你需要在C程序中使用正则表达式,可以考虑链接这些库,并使用它们提供的API来进行查找、替换等工作。例如,使用PCRE库,你可以通过`pcre.h`头文件包含相应的函数,像`pcre_compile()`用于编译正则表达式,`pcre_exec()`用于在给定的输入上执行匹配等。
```c
#include <stdio.h>
#include <pcre.h>
int main() {
const char *regex_str = "(\\w+)";
PCRE *compiled_regex;
char input[] = "This is a test string";
int rc;
compiled_regex = pcre_compile(regex_str, 0, &error_msg, &error_code, NULL);
if (error_code == 0) {
rc = pcre_exec(compiled_regex, NULL, input, sizeof(input), 0, 0, match_buffer, sizeof(match_buffer));
if (rc > 0) {
printf("Match found: %s\n", match_buffer);
}
} else {
printf("Error in compiling regex: %s\n", error_msg);
}
// Don't forget to free the resources
pcre_free(compiled_regex);
return 0;
}
```
这里只是一个基本的例子,实际使用时可能需要处理更复杂的情况,并处理错误和内存管理。
阅读全文
相关推荐
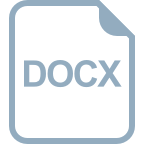
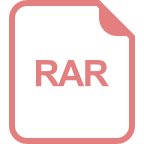
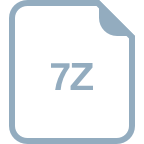
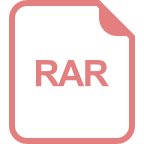
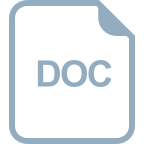
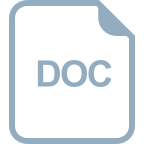
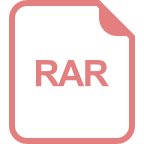
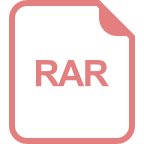
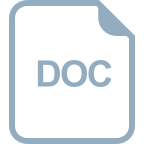
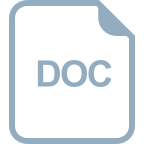



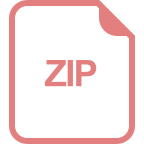
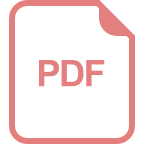
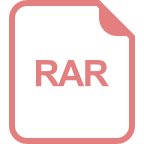
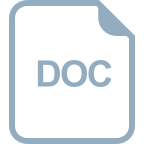