为我提供比较好的代码
时间: 2024-02-15 10:06:12 浏览: 21
好的,以下是一个使用 JavaScript、CSS 和 HTML5 实现的简单贪吃蛇游戏的示例代码,你可以参考一下:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>贪吃蛇游戏</title>
<style>
#game-board {
border: 1px solid black;
width: 600px;
height: 400px;
position: relative;
}
.snake {
width: 20px;
height: 20px;
background-color: green;
position: absolute;
}
#food {
width: 20px;
height: 20px;
background-color: red;
position: absolute;
}
</style>
</head>
<body>
<h1>贪吃蛇游戏</h1>
<div id="game-board"></div>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript 代码:
```javascript
const gameBoard = document.getElementById('game-board');
const snake = [{x: 10, y: 10}];
let food = {x: 0, y: 0};
let dx = 0, dy = 0;
let score = 0;
function drawSnake() {
for (let i = 0; i < snake.length; i++) {
const snakeElement = document.createElement('div');
snakeElement.classList.add('snake');
snakeElement.style.left = snake[i].x * 20 + 'px';
snakeElement.style.top = snake[i].y * 20 + 'px';
gameBoard.appendChild(snakeElement);
}
}
function drawFood() {
const foodElement = document.createElement('div');
foodElement.id = 'food';
foodElement.style.left = food.x * 20 + 'px';
foodElement.style.top = food.y * 20 + 'px';
gameBoard.appendChild(foodElement);
}
function moveSnake() {
const newHead = {x: snake[0].x + dx, y: snake[0].y + dy};
snake.unshift(newHead);
if (newHead.x === food.x && newHead.y === food.y) {
score++;
document.getElementById('score').textContent = score;
generateFood();
} else {
snake.pop();
}
checkCollision();
}
function generateFood() {
food.x = Math.floor(Math.random() * 30);
food.y = Math.floor(Math.random() * 20);
drawFood();
}
function checkCollision() {
const head = snake[0];
if (head.x < 0 || head.x >= 30 || head.y < 0 || head.y >= 20) {
gameOver();
}
for (let i = 1; i < snake.length; i++) {
if (head.x === snake[i].x && head.y === snake[i].y) {
gameOver();
}
}
}
function gameOver() {
clearInterval(intervalId);
alert('游戏结束,得分为:' + score);
}
generateFood();
drawSnake();
const intervalId = setInterval(() => {
gameBoard.innerHTML = '';
moveSnake();
drawSnake();
}, 200);
document.addEventListener('keydown', event => {
switch (event.key) {
case 'ArrowUp':
dx = 0;
dy = -1;
break;
case 'ArrowDown':
dx = 0;
dy = 1;
break;
case 'ArrowLeft':
dx = -1;
dy = 0;
break;
case 'ArrowRight':
dx = 1;
dy = 0;
break;
}
});
```
这段代码实现了一个基本的贪吃蛇游戏,包括蛇的移动、食物的生成、得分的计算、碰撞检测等功能。你可以根据自己的需求进行修改和扩展。
相关推荐
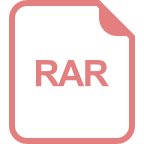
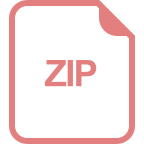
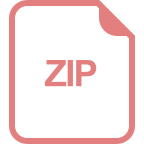















