java grpc_Java + gRPC + grpc-gateway 的实践
时间: 2023-07-08 09:40:35 浏览: 142
Java + gRPC + grpc-gateway 的实践主要分为以下几个步骤:
1. 定义 proto 文件
在 proto 文件中定义需要调用的服务以及方法,同时指定请求和响应的数据类型。例如:
```
syntax = "proto3";
package example;
service ExampleService {
rpc ExampleMethod (ExampleRequest) returns (ExampleResponse) {}
}
message ExampleRequest {
string example_field = 1;
}
message ExampleResponse {
string example_field = 1;
}
```
2. 使用 protoc 编译 proto 文件
使用 protoc 编译 proto 文件,生成 Java 代码。例如:
```
protoc --java_out=./src/main/java ./example.proto
```
3. 实现 gRPC 服务
在 Java 代码中实现定义的 gRPC 服务,例如:
```
public class ExampleServiceImpl extends ExampleServiceGrpc.ExampleServiceImplBase {
@Override
public void exampleMethod(ExampleRequest request, StreamObserver<ExampleResponse> responseObserver) {
// 实现具体逻辑
ExampleResponse response = ExampleResponse.newBuilder().setExampleField("example").build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
```
4. 启动 gRPC 服务器
使用 gRPC 提供的 ServerBuilder 构建 gRPC 服务器,并启动服务器。例如:
```
Server server = ServerBuilder.forPort(8080).addService(new ExampleServiceImpl()).build();
server.start();
```
5. 集成 grpc-gateway
使用 grpc-gateway 可以将 gRPC 服务转换为 HTTP/JSON API。在 proto 文件中添加以下内容:
```
import "google/api/annotations.proto";
service ExampleService {
rpc ExampleMethod (ExampleRequest) returns (ExampleResponse) {
option (google.api.http) = {
post: "/example"
body: "*"
};
}
}
```
在 Java 代码中添加以下内容:
```
Server httpServer = ServerBuilder.forPort(8081).addService(new ExampleServiceImpl()).build();
httpServer.start();
String grpcServerUrl = "localhost:8080";
String httpServerUrl = "localhost:8081";
ProxyServerConfig proxyConfig = new ProxyServerConfig(grpcServerUrl, httpServerUrl, "/example");
HttpProxyServer httpProxyServer = new HttpProxyServer(proxyConfig);
httpProxyServer.start();
```
6. 测试
使用 HTTP/JSON API 调用 gRPC 服务,例如:
```
POST http://localhost:8081/example
Content-Type: application/json
{
"example_field": "example"
}
```
以上就是 Java + gRPC + grpc-gateway 的实践步骤。
相关推荐
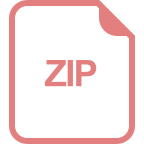
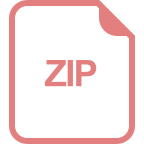














