用c语言with open('alpha.txt', 'r') as f: # 读取文件内容 content = f.read()# 将小写字母转换为大写字母content_upper = content.upper()with open('alpha.txt', 'a') as f: # 将转换后的内容追加到文件中 f.write(content_upper)
时间: 2023-12-09 08:07:04 浏览: 83
在 C 语言中,可以使用标准库中的 `fopen`、`fread`、`fwrite` 和 `fclose` 等函数来实现文件的读写操作。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
FILE *fp;
char c;
// 打开文件
fp = fopen("alpha.txt", "r+");
// 读取并转换文件内容
while ((c = fgetc(fp)) != EOF) {
if (islower(c)) {
c = toupper(c);
// 将转换后的字符追加到文件末尾
fseek(fp, 0, SEEK_END);
fputc(c, fp);
}
}
// 关闭文件
fclose(fp);
return 0;
}
```
需要注意的是,在 C 语言中,文件的读写操作需要谨慎处理文件指针的位置。在示例代码中,为了实现将转换后的字符追加到文件末尾的功能,我们使用了 `fseek(fp, 0, SEEK_END)` 将文件指针移动到文件末尾。
阅读全文
相关推荐
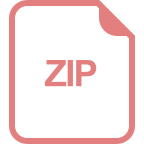
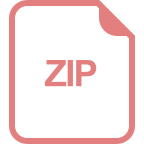
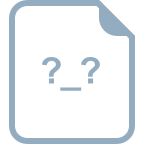
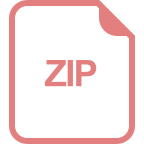
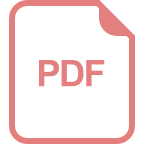
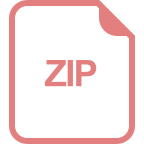
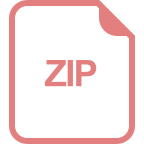
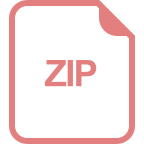
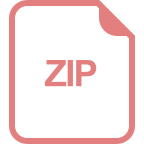
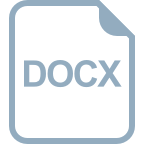
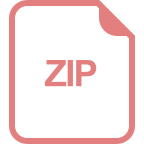
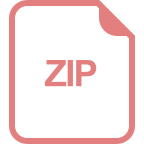