在Vue3中使用DeepAi来实现自动补图
时间: 2024-09-06 15:07:58 浏览: 56
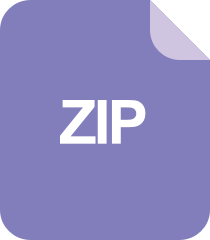
vue3-highcharts:Highcharts.js的Vue 3组件包装器
在 Vue3 中,要利用 DeepAI 实现自动补图功能,你需要结合其 API(如 DeepArt、DeepDream 等)以及 Axios 或者 Vue 的内置 HTTP 客户端来进行网络请求。以下是一个简化的步骤说明:
1. **安装依赖**:首先,需要安装 `axios` 或者 `@vue/cli-plugin-http`,以便在项目中发送 HTTP 请求到 DeepAI 的 API。
```bash
npm install axios
# 或者如果是使用 Vue CLI 的 http 插件
vue add http
```
2. **引入库**:在你的 Vue 组件中导入 Axios 或 VueHTTP,并创建一个实例以便发送 POST 请求。
```javascript
import axios from 'axios';
const api = axios.create({
baseURL: 'https://api.deepai.org/api', // 替换为你实际使用的 DeepAI API URL
headers: {'Content-Type': 'application/json'}, // 设置默认的请求头
});
```
3. **编写方法**:创建一个函数,用于处理图片上传并发送请求给 DeepAI,然后接收返回的处理后的图片数据。
```javascript
async function autoCompleteImage(imageData) {
try {
const response = await api.post('image/complete', {
image: imageData,
style: 'vangogh', // 根据需求选择风格,如 van_gogh, monet等
});
return response.data.result;
} catch (error) {
console.error('Error:', error);
return null;
}
}
```
4. **组件内部使用**:在你的组件里,当用户上传图片或者触发补图逻辑时,调用这个方法并将结果展示出来。
```html
<input type="file" @change="uploadImageForCompletion">
<img :src="completedImage" v-if="completedImage" />
```
```javascript
methods: {
uploadImageForCompletion(e) {
if (e.target.files && e.target.files[0]) {
const reader = new FileReader();
reader.readAsDataURL(e.target.files[0]);
reader.onload = () => {
this.completedImage = await autoCompleteImage(reader.result);
};
}
},
},
```
阅读全文
相关推荐
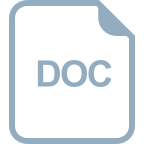
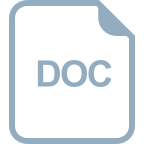
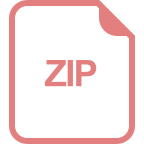
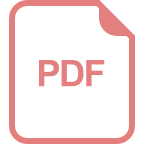
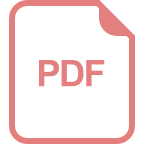
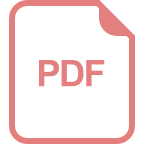
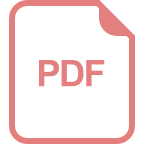
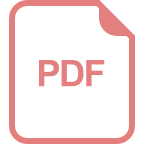
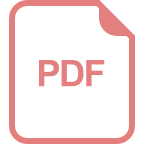
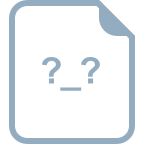
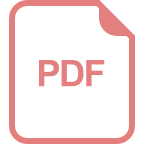
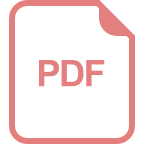
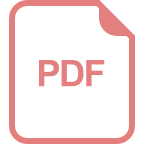
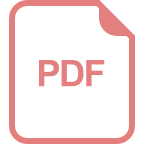
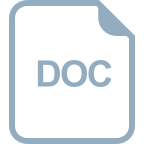
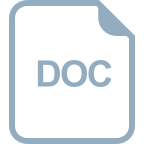