如何使用fgets函数代替scanf函数。
时间: 2023-12-26 19:04:26 浏览: 94
使用fgets函数代替scanf函数的步骤如下:
1. 定义一个字符数组用于存储用户输入的字符串,数组大小要足够大,以免输入的字符串超出数组范围。
```
char input[100];
```
2. 使用fgets函数读取用户输入的字符串,fgets函数的第一个参数是字符数组,第二个参数是数组大小(包括了字符串结束符),第三个参数是标准输入流(通常是stdin)。
```
fgets(input, sizeof(input), stdin);
```
3. 如果fgets函数读取成功,会返回指向输入字符串的指针,否则返回空指针。可以通过判断返回值是否为空指针来检查读取是否成功。如果读取成功,输入的字符串会以换行符结尾,可以使用strchr函数将其替换为字符串结束符。
```
if (fgets(input, sizeof(input), stdin) != NULL) {
char *newline = strchr(input, '\n');
if (newline != NULL) {
*newline = '\0';
}
}
```
4. 可以使用sscanf函数将字符串转换为需要的类型,sscanf函数的第一个参数是输入字符串,第二个参数是格式化字符串,后面的参数是需要转换的变量。可以根据需要进行错误处理。
```
int n;
if (sscanf(input, "%d", &n) == 1) {
printf("你输入的数字是:%d\n", n);
} else {
printf("输入格式不正确!\n");
}
```
完整的代码如下:
```
#include <stdio.h>
#include <string.h>
int main() {
char input[100];
printf("请输入一个数字:");
if (fgets(input, sizeof(input), stdin) != NULL) {
char *newline = strchr(input, '\n');
if (newline != NULL) {
*newline = '\0';
}
int n;
if (sscanf(input, "%d", &n) == 1) {
printf("你输入的数字是:%d\n", n);
} else {
printf("输入格式不正确!\n");
}
}
return 0;
}
```
注意:fgets函数读取的字符串结尾会包含一个换行符,需要将其替换为字符串结束符。
阅读全文
相关推荐
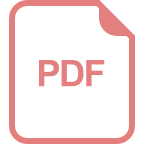
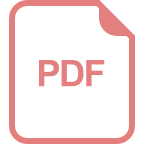















