c# 原生sql快速导出数据到excel
时间: 2023-10-23 13:11:48 浏览: 102
你可以使用 C# 中的 ADO.NET 来快速导出数据到 Excel。下面是一个基本的示例:
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
using System.IO;
using System.Reflection;
using Microsoft.Office.Interop.Excel;
namespace ExportToExcel
{
class Program
{
static void Main(string[] args)
{
// 数据库连接字符串
string connectionString = "Data Source=(local);Initial Catalog=MyDatabase;Integrated Security=True";
// SQL 查询语句
string query = "SELECT * FROM MyTable";
// 创建 SQL 连接对象
using (SqlConnection connection = new SqlConnection(connectionString))
{
// 打开连接
connection.Open();
// 创建 SQL 命令对象
using (SqlCommand command = new SqlCommand(query, connection))
{
// 创建数据适配器对象
using (SqlDataAdapter adapter = new SqlDataAdapter(command))
{
// 创建数据集对象
DataSet dataSet = new DataSet();
// 填充数据集
adapter.Fill(dataSet);
// 创建 Excel 应用程序对象
Application excel = new Application();
excel.Visible = false;
excel.DisplayAlerts = false;
// 创建 Excel 工作簿对象
Workbook workbook = excel.Workbooks.Add(Missing.Value);
// 创建 Excel 工作表对象
Worksheet worksheet = (Worksheet)workbook.ActiveSheet;
// 写入数据到 Excel 工作表
for (int i = 0; i < dataSet.Tables[0].Columns.Count; i++)
{
worksheet.Cells[1, i + 1] = dataSet.Tables[0].Columns[i].ColumnName;
}
for (int i = 0; i < dataSet.Tables[0].Rows.Count; i++)
{
for (int j = 0; j < dataSet.Tables[0].Columns.Count; j++)
{
worksheet.Cells[i + 2, j + 1] = dataSet.Tables[0].Rows[i][j];
}
}
// 保存 Excel 文件
workbook.SaveAs("data.xlsx");
// 关闭 Excel 应用程序对象
excel.Quit();
}
}
}
}
}
}
```
在上面的代码中,我们首先定义了数据库连接字符串和 SQL 查询语句。然后,我们使用 ADO.NET 创建 SQL 连接对象、SQL 命令对象和数据适配器对象,使用数据适配器对象填充数据集。接着,我们创建 Excel 应用程序对象、Excel 工作簿对象和 Excel 工作表对象,然后将数据写入 Excel 工作表。最后,我们保存 Excel 文件并关闭 Excel 应用程序对象。
阅读全文
相关推荐
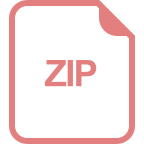
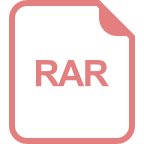


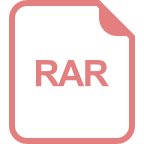
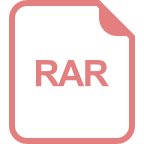
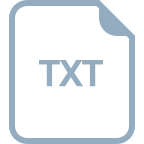
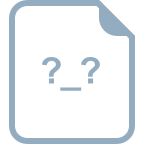
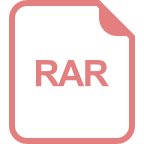
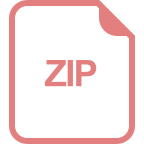
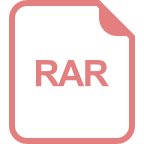
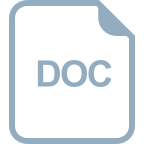
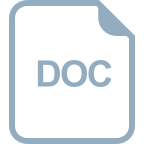
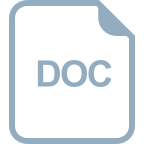
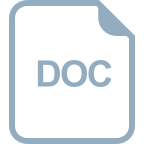
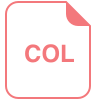
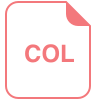