基于vue创建一个子组件,然后将父组件的属性msg:“hello wolrd”的值在子组件中显示!
时间: 2024-05-15 22:17:11 浏览: 40
好的,这个问题比较简单,可以直接回答。在子组件的模板中,可以使用{{msg}}来引用父组件传递过来的属性值,代码如下:
```
// 子组件模板代码
<template>
<div>{{msg}}</div>
</template>
// 父组件模板代码
<template>
<div>
<child-component :msg="'hello world'"></child-component>
</div>
</template>
// 父组件中通过props传递属性
export default {
components: {
ChildComponent,
},
};
```
希望我的回答可以帮助到您。
相关问题
vue子组件如何给父组件传一个值然后在父组件上显示
可以使用 props 将子组件的数据传递给父组件。在子组件中,需要将需要传递的数据作为一个属性绑定到组件的标签上,例如:
```html
<template>
<div>
<button @click="handleClick">点击传递数据</button>
</div>
</template>
<script>
export default {
methods: {
handleClick() {
// 将数据作为事件参数传递给父组件
this.$emit('receiveData', 'hello world');
}
}
}
</script>
```
在父组件中,可以通过监听子组件触发的事件来获取传递的数据:
```html
<template>
<div>
<child-component @receiveData="handleReceiveData"></child-component>
<p v-if="data">{{data}}</p>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
data: null // 父组件中要显示的子组件传递的数据
}
},
methods: {
handleReceiveData(data) {
// 监听子组件的事件,获取传递的数据
this.data = data;
}
}
}
</script>
```
上面的代码中,子组件中定义了 handleClick 方法,当点击按钮时,会通过 $emit 方法触发子组件的 receiveData 事件,并将数据作为参数传递给父组件。在父组件中,通过监听子组件触发的 receiveData 事件,获取传递的数据,然后在页面上显示出来。
vue代码示例父组件如何给子组件传一个值然后在父组件上显示
可以通过props将值传递给子组件,然后在子组件中使用该值。示例代码如下:
父组件:
<template>
<div>
<child-component :message="msg"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent'
export default {
components: {
ChildComponent
},
data () {
return {
msg: 'hello'
}
}
}
</script>
子组件:
<template>
<div>
{{ message }}
</div>
</template>
<script>
export default {
props: ['message']
}
</script>
这样,父组件中的msg值就可以传递给子组件,并在子组件中使用。
阅读全文
相关推荐
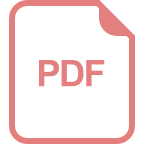
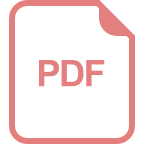
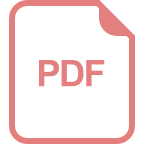













