通讯录管理系统c++类
时间: 2023-09-21 21:11:35 浏览: 74
以下是一个简单的通讯录管理系统的C++类:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Contact {
public:
Contact(string name, string phone) : name_(name), phone_(phone) {}
string getName() const {
return name_;
}
string getPhone() const {
return phone_;
}
private:
string name_;
string phone_;
};
class ContactList {
public:
void addContact(string name, string phone) {
Contact contact(name, phone);
contacts_.push_back(contact);
}
void removeContact(string name) {
for (auto it = contacts_.begin(); it != contacts_.end(); it++) {
if (it->getName() == name) {
contacts_.erase(it);
break;
}
}
}
void printContacts() const {
cout << "Contacts:" << endl;
for (const auto& contact : contacts_) {
cout << contact.getName() << " - " << contact.getPhone() << endl;
}
}
private:
vector<Contact> contacts_;
};
int main() {
ContactList contactList;
contactList.addContact("Alice", "123-456-7890");
contactList.addContact("Bob", "234-567-8901");
contactList.printContacts();
contactList.removeContact("Alice");
contactList.printContacts();
return 0;
}
```
这个类使用了C++的STL库中的vector来存储联系人列表。Contact类表示一个联系人,包含姓名和电话号码。ContactList类表示联系人列表,提供添加和删除联系人的方法,以及打印联系人列表的方法。在main函数中,我们创建了一个ContactList对象,添加了两个联系人,然后打印联系人列表。接着,我们删除了一个联系人,并再次打印联系人列表。
相关推荐
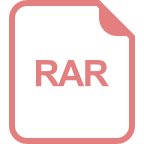
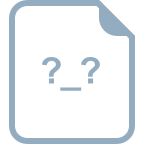














