c语言中怎么实现
时间: 2023-07-02 07:14:25 浏览: 103
好的,如果您想要在 C 语言中实现统计自定义函数个数并计算平均行数的功能,可以参考下面的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024 // 最大行长度
#define MAX_FUNC_NUM 100 // 最大函数数
int count_lines(char *filename, int start_line, int end_line);
int match_func(char *line, char *func_name, int *func_start);
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <filename>\n", argv[0]);
exit(1);
}
char *filename = argv[1];
FILE *fp = fopen(filename, "r");
if (!fp) {
printf("Failed to open file %s\n", filename);
exit(1);
}
char line[MAX_LINE_LEN];
char func_name[MAX_LINE_LEN];
int func_start = 0;
int func_lines[MAX_FUNC_NUM];
int func_count = 0;
int total_lines = 0;
while (fgets(line, MAX_LINE_LEN, fp)) {
int len = strlen(line);
if (len > 0 && line[len - 1] == '\n') {
line[len - 1] = '\0'; // 去掉行末的换行符
}
if (match_func(line, func_name, &func_start)) {
// 匹配到函数名,记录函数开始行号
func_lines[func_count] = func_start;
func_count++;
continue;
}
total_lines++;
}
fclose(fp);
// 计算各个函数的行数
int func_total_lines = 0;
for (int i = 0; i < func_count; i++) {
int start_line = func_lines[i];
int end_line = start_line;
while (end_line < total_lines && !match_func(line, func_name, &func_start)) {
end_line++;
fgets(line, MAX_LINE_LEN, fp);
}
func_total_lines += count_lines(filename, start_line, end_line - 1);
}
// 计算平均行数
double avg_lines = func_count > 0 ? (double)func_total_lines / func_count : 0;
printf("Total functions: %d\n", func_count);
printf("Average lines per function: %.2f\n", avg_lines);
return 0;
}
// 计算文件中特定行号范围内的代码行数
int count_lines(char *filename, int start_line, int end_line) {
FILE *fp = fopen(filename, "r");
if (!fp) {
printf("Failed to open file %s\n", filename);
exit(1);
}
int line_num = 1;
int line_count = 0;
char line[MAX_LINE_LEN];
while (fgets(line, MAX_LINE_LEN, fp)) {
if (line_num >= start_line && line_num <= end_line) {
// 如果当前行在范围内,统计代码行数
int len = strlen(line);
int is_code_line = 0;
for (int i = 0; i < len; i++) {
if (line[i] != ' ' && line[i] != '\t' && line[i] != '\r' && line[i] != '\n') {
is_code_line = 1;
break;
}
}
if (is_code_line) {
line_count++;
}
}
line_num++;
}
fclose(fp);
return line_count;
}
// 判断当前行是否为函数定义行,如果是,返回函数名和函数开始行号
int match_func(char *line, char *func_name, int *func_start) {
int len = strlen(line);
if (len < 3 || line[len - 2] != ')' || line[len - 1] != '{') {
// 不是函数定义行
return 0;
}
// 去掉行末的 '{' 符号
line[len - 1] = '\0';
// 查找函数名
int pos = len - 3;
while (pos >= 0 && line[pos] != ' ') {
pos--;
}
strcpy(func_name, line + pos + 1);
// 记录函数开始行号
*func_start = 1;
fseek(stdin, 0, SEEK_SET);
char buf[MAX_LINE_LEN];
while (fgets(buf, MAX_LINE_LEN, stdin)) {
if (strcmp(buf, line) == 0) {
break;
}
(*func_start)++;
}
return 1;
}
```
这个程序会读取指定的 C 语言代码文件,统计其中的自定义函数个数,并计算出这些函数的平均行数。具体实现的思路和 Python 版本类似,不同之处在于 C 语言需要手动读取文件和处理字符串,需要更多的细节处理。程序中使用了一些 C 语言的库函数,比如 fgets() 和 strcmp(),如果您不熟悉这些函数的使用,可以参考 C 语言的相关教程。
阅读全文
相关推荐


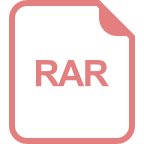
