使用C语言,完成下列题目。 设ha=(a1,a2,…,an)和 hb=(b1,b2,…,bm)是两个带头结点的循环单链表,ha和hb是尾指针,分别指向ha和hb两个循环单链表中的最后一个结点,设计一个算法将这两个表合并为带头结点的循环单链表hc,hc是尾指针。生成完整c语言代码
时间: 2024-11-12 12:46:11 浏览: 25
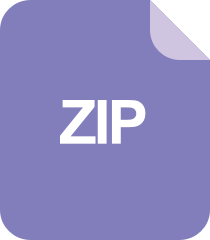
c语言吃逗游戏源码===C语言小游戏源码
在C语言中,合并两个循环单链表可以采用遍历两个列表并逐个添加元素到新链表的方式。这里是一个简单的示例代码,假设`Node`结构体表示链表节点,包含数据域`data`和指向下一个节点的指针`next`:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新的链表节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = data;
newNode->next = newNode; // 循环链接,因为是尾部链接
return newNode;
}
// 合并两个循环链表
Node* mergeTwoLists(Node* ha, Node* hb) {
if (ha == NULL) return hb;
if (hb == NULL) return ha;
Node* hc = createNode(ha->data); // 将ha的第一个元素添加到新链表
Node* tempHc = hc, *tempHa = ha, *tempBb = hb;
while (true) {
tempHc->next = tempBb->next;
if (tempBb->next == hb) {
tempBb->next = tempHa->next;
break; // 如果hb到达尾部,则ha的下一个节点连接到尾部
} else {
tempHa->next = tempBb->next;
tempHa = tempHa->next;
tempBb = tempBb->next;
}
// 继续添加ha和hb的下一个元素,直到其中一个链表结束
tempHc = tempHc->next;
}
return hc;
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp != temp->next) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
// 为示例创建两个循环链表
Node* ha = createNode(1);
ha->next = createNode(2);
ha->next->next = ha; // 设置循环
Node* hb = createNode(3);
hb->next = createNode(4);
hb->next->next = hb; // 设置循环
Node* mergedList = mergeTwoLists(ha, hb);
printf("Merged list: ");
printList(mergedList);
return 0;
}
```
注意:这个代码片段演示了如何合并两个循环链表的基本操作,实际应用中需要考虑错误处理和内存管理。
阅读全文
相关推荐
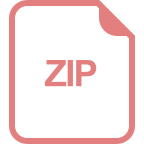
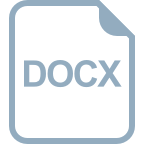
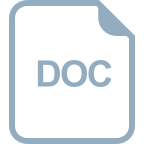
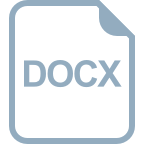
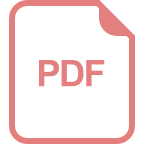
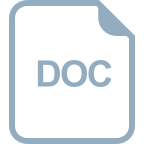
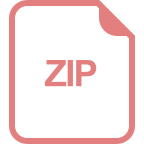
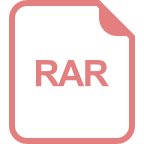
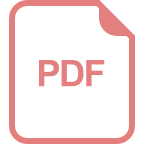
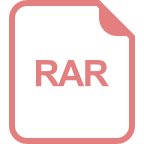
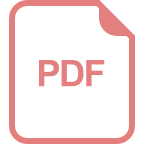
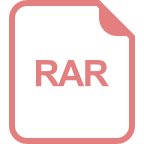