如何在Java中通过网络调用远程服务器上的shell脚本,但不使用ssh2库或jsch库实现?
时间: 2024-12-26 18:30:26 浏览: 8
在Java中,如果你想通过网络调用远程服务器上的shell脚本但不使用专门的SSH库,比如ssh2或jsch,你可以考虑使用HTTP请求或Socket编程结合Java的Runtime类或者ProcessBuilder类来间接实现这个目标。以下是一个基本的步骤:
1. **使用HTTP POST**: 将shell脚本的内容作为POST数据发送到一个支持接收并执行脚本的服务端口,例如Python的Flask应用或Node.js的Express应用。服务器接收到请求后,可以解析脚本,然后在后台执行。
```java
URL url = new URL("http://yourserver.com/run-script");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "text/plain");
connection.setDoOutput(true);
try (OutputStream os = connection.getOutputStream()) {
os.write(shellScript.getBytes(StandardCharsets.UTF_8));
}
int responseCode = connection.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder output = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
output.append(inputLine);
}
in.close();
```
2. **使用Socket连接**: 连接到服务器,然后向其发送shell命令。服务器接收到命令后,通过os.system()或者其他类似的方式执行脚本,并将结果返回给客户端。
```java
Socket socket = new Socket("your-server-ip", yourServerPort);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out.println(shellCommand);
String result = "";
while ((result = in.readLine()) != null) {
// process the server's response
}
socket.close();
```
请注意,这种方法通常安全性较差,因为直接暴露了shell执行权限。对于生产环境,推荐使用安全的SSH协议。
阅读全文
相关推荐
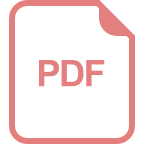
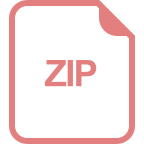
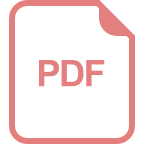
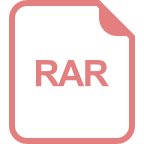
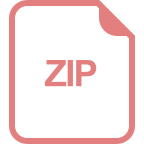
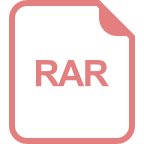
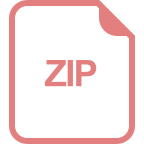
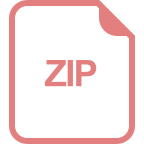
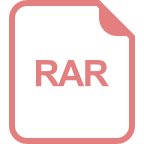
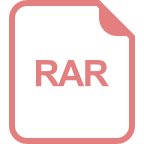
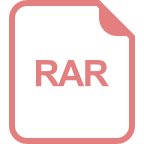
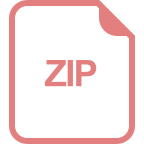
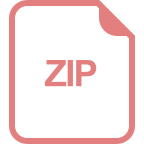
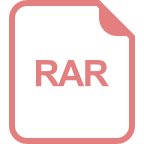
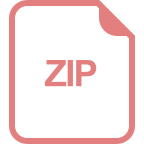
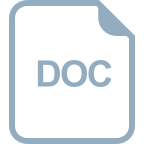
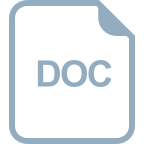
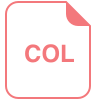
