android studio案例代码: 1.使用相对布局描绘UI界面; 2.分别点击三个按钮,每点击一个按钮会切换相应的照片
时间: 2024-10-18 18:18:02 浏览: 34
在Android Studio中创建一个简单的案例,我们将使用相对布局(Relative Layout)来设计用户界面,并通过监听按钮点击事件来切换显示的照片。首先,你需要新建一个`activity_main.xml`文件来放置UI元素:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/picture1" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="按钮1"
android:layout_below="@id/imageView"
android:layout_centerHorizontal="true" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="按钮2"
android:layout_below="@id/button1"
android:layout_centerHorizontal="true" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="按钮3"
android:layout_below="@id/button2"
android:layout_centerHorizontal="true" />
</RelativeLayout>
```
接下来,在`MainActivity.java`中,我们需要处理按钮的点击事件和图片的切换:
```java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private Button button1, button2, button3;
private int currentPhotoIndex = 0;
private String[] photoNames = {"picture1", "picture2", "picture3"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.imageView);
button1 = findViewById(R.id.button1);
button2 = findViewById(R.id.button2);
button3 = findViewById(R.id.button3);
// 设置初始图片
imageView.setImageResource(getResourceId(photoNames[currentPhotoIndex]));
// 给每个按钮添加点击监听
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
switchPhotos();
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
switchPhotos();
}
});
button3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
switchPhotos();
}
});
}
private void switchPhotos() {
currentPhotoIndex++;
if (currentPhotoIndex >= photoNames.length) {
currentPhotoIndex = 0;
}
imageView.setImageResource(getResourceId(photoNames[currentPhotoIndex]));
}
private int getResourceId(String resourceName) {
return getResources().getIdentifier(resourceName, "drawable", getPackageName());
}
}
```
在这个例子中,当你点击任一按钮时,都会调用`switchPhotos()`方法,改变`imageView`显示的图片。
阅读全文
相关推荐
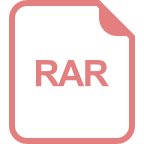
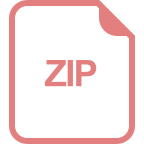
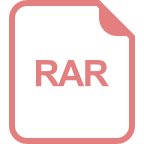
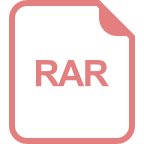
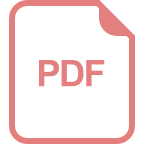
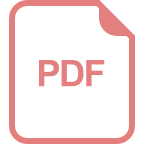
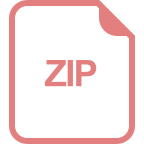
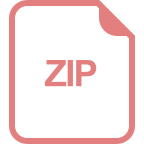
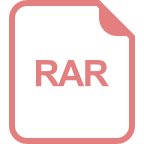
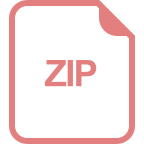
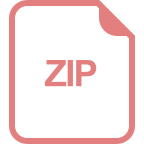
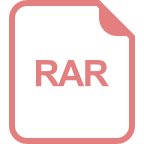
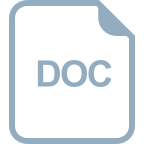
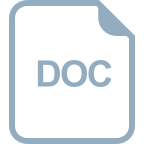
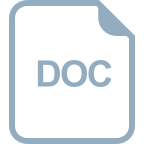
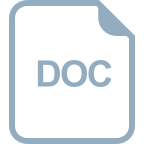
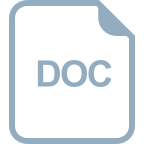
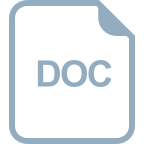