在一个 java 类中,怎么引用一个 bean 中的方法
时间: 2024-09-12 20:17:35 浏览: 34
在 Java 中,如果你想要在类中引用另一个 Bean(通常是一个 Spring 管理的 Bean)中的方法,可以按照以下步骤操作:
1. **Spring 注入**: 使用 `@Autowired` 或者 `@Qualifier` 注解将依赖注入到你的类中。假设你要注入的方法在一个名为 `OtherService` 的 Bean 中:
```java
import org.springframework.beans.factory.annotation.Autowired;
public class MyClass {
@Autowired
private OtherService otherService; // 假设 OtherService 是一个实现了某个接口的服务
// 如果 OtherService 中有一个公开的方法,如名为 "myMethod" 的方法
public void callOtherServiceMethod() {
// 调用 OtherService 的方法
String result = otherService.myMethod();
System.out.println(result);
}
}
```
2. **基于接口调用**: 如果你想通过接口而不是具体实现调用方法,确保注入的是接口的实例:
```java
public class MyClass {
private IOtherService otherService; // 假设 IOtherService 是 OtherService 的接口
@Autowired
public void setOtherService(IOtherService service) {
this.otherService = service;
}
// 接口方法的调用
public void callMethodThroughInterface() {
String result = otherService.myMethod();
System.out.println(result);
}
}
```
3. **Spring Expression Language (SpEL)**: 如果你需要在运行时动态调用,可以使用 SpEL 表达式:
```java
public class MyClass {
private OtherService otherService;
// SpEL 示例
public void callMethodUsingSpEL(String methodName) {
Object result = Beans.getExpressionResolver().getValue(otherService, "#{otherService.''" + methodName + "'()}");
System.out.println(result);
}
}
```
阅读全文
相关推荐
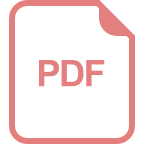
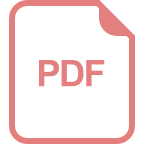
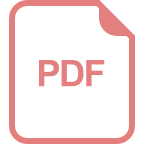
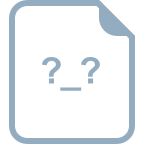
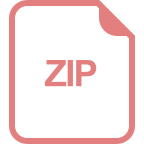
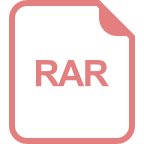
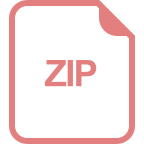
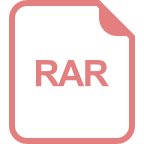
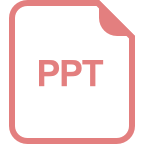
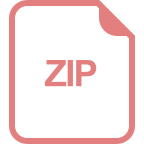
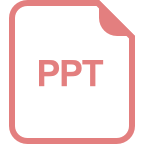
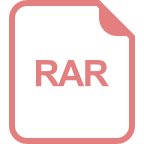
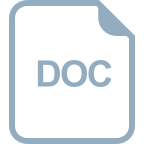
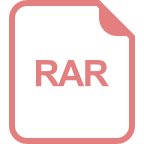