java调用python算法
时间: 2023-10-12 07:48:14 浏览: 38
要在Java中调用Python算法,你可以使用Java的ProcessBuilder类启动一个Python进程,并通过输入输出流与该进程进行交互。下面是一个简单的示例:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class PythonCaller {
public static void main(String[] args) {
try {
// 构建Python进程命令
ProcessBuilder pb = new ProcessBuilder("python", "path/to/your/python/script.py");
Process process = pb.start();
// 获取Python进程的输入输出流
BufferedReader inputReader = new BufferedReader(new InputStreamReader(process.getInputStream()));
BufferedReader errorReader = new BufferedReader(new InputStreamReader(process.getErrorStream()));
// 向Python进程发送输入
process.getOutputStream().write("input_data".getBytes());
process.getOutputStream().flush();
// 读取Python进程的输出
String line;
while ((line = inputReader.readLine()) != null) {
System.out.println(line);
}
// 读取Python进程的错误输出
while ((line = errorReader.readLine()) != null) {
System.err.println(line);
}
// 等待Python进程执行完毕
int exitCode = process.waitFor();
System.out.println("Python process exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,你需要将`"path/to/your/python/script.py"`替换为你实际的Python脚本路径。你可以通过`process.getOutputStream().write()`向Python进程发送输入数据,并通过`inputReader.readLine()`读取Python进程的输出结果。
请注意,这只是一个简单的示例,实际情况可能更复杂。你可能还需要处理Python进程的错误输出、异常情况等。另外,确保你的Java环境中已经安装了Python,并且Python脚本的依赖库已安装。
相关推荐
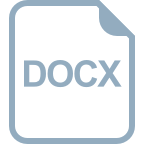
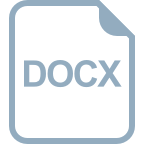
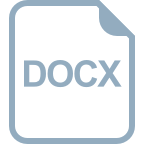














