帮我设计一个ssm留言板,要求两张表 评论和回复表,且回复没有限制,全查且返回格式为树状列表结构
时间: 2024-03-14 11:48:23 浏览: 197
好的,我可以为您提供一个包含评论和回复表的SSM留言板的设计思路:
1. 数据库设计:
评论表comment:
- id: 评论id
- content: 评论内容
- create_time: 评论时间
回复表reply:
- id: 回复id
- content: 回复内容
- create_time: 回复时间
- comment_id: 评论id,表示该回复属于哪个评论
- parent_id: 父回复id,如果没有父回复,则为0
2. Java代码实现:
Comment类:
```
public class Comment {
private int id;
private String content;
private Date createTime;
private List<Reply> replies;
// getter and setter
}
```
Reply类:
```
public class Reply {
private int id;
private String content;
private Date createTime;
private int commentId;
private int parentId;
private List<Reply> children;
// getter and setter
}
```
CommentMapper接口:
```
public interface CommentMapper {
/**
* 获取所有评论
*/
List<Comment> getAllComments();
/**
* 根据id获取评论
*/
Comment getCommentById(int id);
/**
* 新增评论
*/
int addComment(Comment comment);
/**
* 获取指定评论的所有回复
*/
List<Reply> getRepliesByCommentId(int commentId);
/**
* 新增回复
*/
int addReply(Reply reply);
}
```
CommentServiceImpl实现类:
```
@Service
public class CommentServiceImpl implements CommentService {
@Autowired
private CommentMapper commentMapper;
/**
* 获取所有评论
*/
@Override
public List<Comment> getAllComments() {
List<Comment> comments = commentMapper.getAllComments();
// 将评论列表转化为树状结构
return buildCommentTree(comments);
}
/**
* 根据id获取评论
*/
@Override
public Comment getCommentById(int id) {
return commentMapper.getCommentById(id);
}
/**
* 新增评论
*/
@Override
public int addComment(Comment comment) {
return commentMapper.addComment(comment);
}
/**
* 获取指定评论的所有回复
*/
@Override
public List<Reply> getRepliesByCommentId(int commentId) {
List<Reply> replies = commentMapper.getRepliesByCommentId(commentId);
// 将回复列表转化为树状结构
return buildReplyTree(replies);
}
/**
* 新增回复
*/
@Override
public int addReply(Reply reply) {
return commentMapper.addReply(reply);
}
/**
* 将评论列表转化为树状结构
*/
private List<Comment> buildCommentTree(List<Comment> comments) {
Map<Integer, Comment> commentMap = new HashMap<>();
for (Comment comment : comments) {
commentMap.put(comment.getId(), comment);
}
List<Comment> roots = new ArrayList<>();
for (Comment comment : comments) {
List<Reply> replies = getRepliesByCommentId(comment.getId());
comment.setReplies(replies);
commentMap.put(comment.getId(), comment);
int parentId = 0;
Comment parentComment = null;
if (replies != null && replies.size() > 0) {
parentId = replies.get(0).getParentId();
parentComment = commentMap.get(parentId);
if (parentComment != null) {
if (parentComment.getReplies() == null) {
parentComment.setReplies(new ArrayList<>());
}
parentComment.getReplies().add(comment);
}
}
if (parentId == 0) {
roots.add(comment);
}
}
return roots;
}
/**
* 将回复列表转化为树状结构
*/
private List<Reply> buildReplyTree(List<Reply> replies) {
Map<Integer, Reply> replyMap = new HashMap<>();
for (Reply reply : replies) {
replyMap.put(reply.getId(), reply);
}
List<Reply> roots = new ArrayList<>();
for (Reply reply : replies) {
int parentId = reply.getParentId();
if (parentId == 0) {
roots.add(reply);
} else {
Reply parent = replyMap.get(parentId);
if (parent != null) {
if (parent.getChildren() == null) {
parent.setChildren(new ArrayList<>());
}
parent.getChildren().add(reply);
}
}
}
return roots;
}
}
```
3. 前端页面实现:
使用递归方式,遍历树状结构渲染页面即可。
以上是一个包含评论和回复表的SSM留言板设计思路,您可以根据实际需求进行修改和优化。
阅读全文
相关推荐
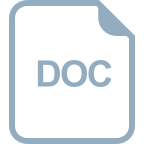
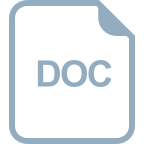
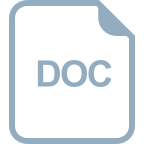
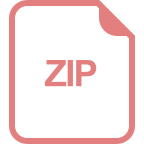
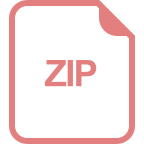
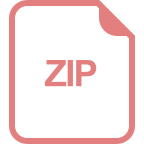
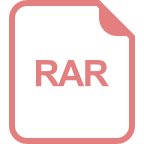
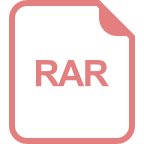
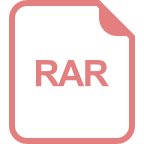
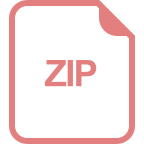
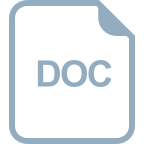
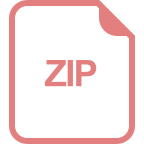
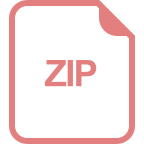
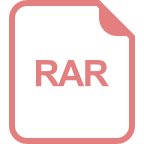
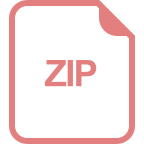
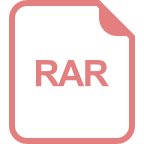
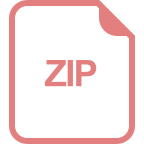
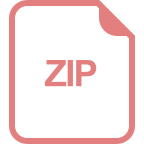