vb.net所以全部文件操作函数实例代码汇总
时间: 2023-08-11 10:06:51 浏览: 65
以下是 VB.NET 中的文件操作函数的完整列表及其示例代码:
1. `File.Exists` - 检查文件是否存在
```
Dim filePath As String = "C:\example.txt"
If File.Exists(filePath) Then
Console.WriteLine("File exists")
Else
Console.WriteLine("File does not exist")
End If
```
2. `File.ReadAllText` - 读取文本文件的内容
```
Dim filePath As String = "C:\example.txt"
Dim fileContents As String = File.ReadAllText(filePath)
Console.WriteLine(fileContents)
```
3. `File.ReadAllLines` - 读取文本文件的所有行
```
Dim filePath As String = "C:\example.txt"
Dim fileLines As String() = File.ReadAllLines(filePath)
For Each line As String In fileLines
Console.WriteLine(line)
Next
```
4. `File.ReadAllText` - 读取二进制文件的内容
```
Dim filePath As String = "C:\example.bin"
Dim fileBytes As Byte() = File.ReadAllBytes(filePath)
For Each b As Byte In fileBytes
Console.Write(b & " ")
Next
```
5. `File.WriteAllText` - 创建一个新文件并向其中写入文本
```
Dim filePath As String = "C:\example.txt"
Dim fileContents As String = "Hello, world!"
File.WriteAllText(filePath, fileContents)
```
6. `File.WriteAllLines` - 创建一个新文件并向其中写入多行文本
```
Dim filePath As String = "C:\example.txt"
Dim fileLines As String() = {"Line 1", "Line 2", "Line 3"}
File.WriteAllLines(filePath, fileLines)
```
7. `File.WriteAllBytes` - 创建一个新文件并向其中写入二进制数据
```
Dim filePath As String = "C:\example.bin"
Dim fileBytes As Byte() = {1, 2, 3, 4, 5}
File.WriteAllBytes(filePath, fileBytes)
```
8. `File.AppendAllText` - 向现有文件中追加文本
```
Dim filePath As String = "C:\example.txt"
Dim fileContents As String = "New line of text"
File.AppendAllText(filePath, fileContents)
```
9. `File.AppendAllLines` - 向现有文件中追加多行文本
```
Dim filePath As String = "C:\example.txt"
Dim fileLines As String() = {"Line 4", "Line 5", "Line 6"}
File.AppendAllLines(filePath, fileLines)
```
10. `File.Copy` - 复制文件
```
Dim sourcePath As String = "C:\example.txt"
Dim destinationPath As String = "C:\example_copy.txt"
File.Copy(sourcePath, destinationPath)
```
11. `File.Move` - 移动文件
```
Dim sourcePath As String = "C:\example.txt"
Dim destinationPath As String = "C:\ExampleFolder\example.txt"
File.Move(sourcePath, destinationPath)
```
12. `File.Delete` - 删除文件
```
Dim filePath As String = "C:\example.txt"
File.Delete(filePath)
```
这些是 VB.NET 中可用的文件操作函数及其示例代码。请注意,这些函数不是全部的文件操作函数,但是它们涵盖了大部分常见的文件操作需求。如果您需要执行更高级的文件操作,请查看 .NET Framework 中其他的文件操作类和函数。
相关推荐
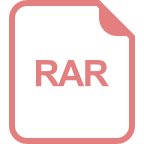














