C语言链表的遍历
时间: 2023-06-28 17:04:25 浏览: 235
遍历链表是指按照一定的顺序访问链表中的每一个节点。C语言中链表的遍历可以通过指针来实现。遍历链表的基本思路是从链表的头节点开始,依次遍历链表中的每一个节点,直到链表的尾节点。以下是一个简单的C语言链表遍历的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void displayList(struct node *head) {
struct node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
}
int main() {
struct node *head = NULL;
struct node *second = NULL;
struct node *third = NULL;
// 分配内存
head = (struct node *)malloc(sizeof(struct node));
second = (struct node *)malloc(sizeof(struct node));
third = (struct node *)malloc(sizeof(struct node));
// 赋值
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
// 遍历链表
displayList(head);
return 0;
}
```
在上面的代码中,我们定义了一个结构体 `node` 作为链表的节点,并且定义了一个函数 `displayList` 来遍历链表。在 `main` 函数中,我们创建了一个链表并赋值,然后调用 `displayList` 函数来遍历链表并输出每个节点的值。
相关推荐
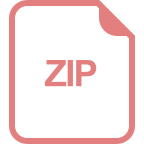
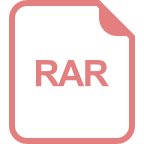
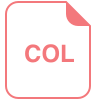
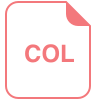
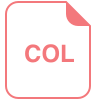
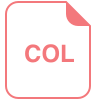
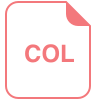









