使用Python实现词频统计及文本向量化
时间: 2024-03-15 13:44:26 浏览: 76
好的,这个问题很简单,我可以为您提供一些代码示例和解释。首先,让我们从词频统计开始。
假设我们有一段文本:
```
text = "This is a sample text that we can use to demonstrate how to perform word frequency analysis in Python."
```
我们可以使用Python中的Counter对象来实现词频统计。Counter是Python的内置模块collections中的一个类,它可以用来计算可迭代对象中每个元素的出现次数。
示例代码如下:
```python
from collections import Counter
text = "This is a sample text that we can use to demonstrate how to perform word frequency analysis in Python."
# 将文本转换为小写并使用split()方法将其拆分为单词列表
word_list = text.lower().split()
# 使用Counter对象计算每个单词的出现次数
word_count = Counter(word_list)
# 打印结果
print(word_count)
```
输出结果:
```
Counter({'to': 2, 'this': 1, 'is': 1, 'a': 1, 'sample': 1, 'text': 1, 'that': 1, 'we': 1, 'can': 1, 'use': 1, 'demonstrate': 1, 'how': 1, 'perform': 1, 'word': 1, 'frequency': 1, 'analysis': 1, 'in': 1, 'python.': 1})
```
接下来,让我们来看一下文本向量化的实现。
文本向量化是将文本转换为数值向量的过程,通常用于机器学习和自然语言处理任务中。最常见的文本向量化方法是词袋模型(Bag of Words),它将文本表示为单词的出现次数或者单词的存在与否。
示例代码如下:
```python
from sklearn.feature_extraction.text import CountVectorizer
text_list = ["This is the first document.", "This is the second document.", "And this is the third one.", "Is this the first document?"]
# 创建CountVectorizer对象并拟合数据
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(text_list)
# 打印输出结果
print(X.toarray())
print(vectorizer.get_feature_names())
```
输出结果:
```
[[0 1 1 1 0 0 0 1]
[0 1 1 0 0 1 0 1]
[1 1 0 0 1 0 1 1]
[0 1 1 1 0 0 0 1]]
['and', 'document', 'first', 'is', 'one', 'second', 'the', 'third']
```
以上代码使用了scikit-learn库中的CountVectorizer类,它可以将文本转换为稀疏矩阵。在这个例子中,我们使用了一个包含4个文本的文本列表,并将它们转换为数值向量。最终得到的向量表示每个文本中单词的出现次数,向量的维度为8,对应着每个单词。
阅读全文
相关推荐
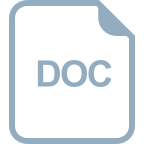
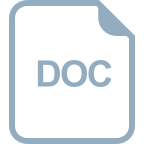
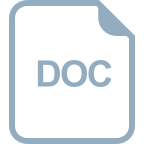


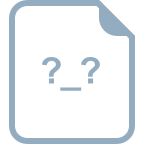
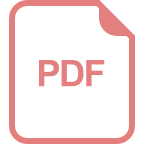
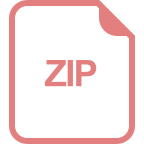
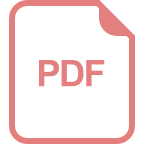
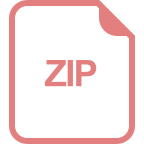
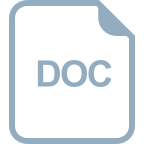
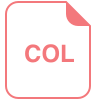
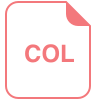




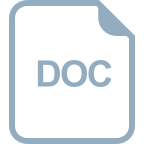