wpf实现步骤条控件代码
时间: 2023-10-26 22:18:43 浏览: 73
以下是一个简单的WPF步骤条控件的示例代码:
StepBar.xaml:
```xaml
<UserControl x:Class="StepBarControl.StepBar"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="30" d:DesignWidth="300">
<ItemsControl Name="Steps" ItemsSource="{Binding}">
<ItemsControl.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding}" Margin="5">
<TextBlock.Style>
<Style TargetType="TextBlock">
<Setter Property="FontWeight" Value="Normal"/>
<Style.Triggers>
<DataTrigger Binding="{Binding ElementName=StepBar, Path=CurrentStep}" Value="{Binding RelativeSource={RelativeSource AncestorType=TextBlock}, Path=Name}">
<Setter Property="FontWeight" Value="Bold"/>
</DataTrigger>
</Style.Triggers>
</Style>
</TextBlock.Style>
</TextBlock>
</DataTemplate>
</ItemsControl.ItemTemplate>
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<StackPanel Orientation="Horizontal"/>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
</ItemsControl>
</UserControl>
```
StepBar.xaml.cs:
```csharp
using System.Collections.ObjectModel;
using System.Windows;
using System.Windows.Controls;
namespace StepBarControl
{
public partial class StepBar : UserControl
{
public static readonly DependencyProperty CurrentStepProperty =
DependencyProperty.Register("CurrentStep", typeof(string), typeof(StepBar),
new PropertyMetadata(string.Empty, OnCurrentStepChanged));
public ObservableCollection<string> Steps
{
get { return (ObservableCollection<string>)GetValue(StepsProperty); }
set { SetValue(StepsProperty, value); }
}
public string CurrentStep
{
get { return (string)GetValue(CurrentStepProperty); }
set { SetValue(CurrentStepProperty, value); }
}
public static readonly DependencyProperty StepsProperty =
DependencyProperty.Register("Steps", typeof(ObservableCollection<string>), typeof(StepBar),
new PropertyMetadata(new ObservableCollection<string>()));
public StepBar()
{
InitializeComponent();
DataContext = Steps;
}
private static void OnCurrentStepChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
var stepBar = (StepBar)d;
foreach (var item in stepBar.Steps)
{
if (item == e.NewValue.ToString())
{
item.FontWeight = FontWeights.Bold;
}
else
{
item.FontWeight = FontWeights.Normal;
}
}
}
private void Steps_MouseDown(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
var textBlock = e.Source as TextBlock;
if (textBlock != null)
{
CurrentStep = textBlock.Text;
}
}
}
}
```
使用步骤:
1. 在你的WPF项目中添加StepBar.xaml和StepBar.xaml.cs文件。
2. 在XAML中引用StepBar控件:
```xaml
<Window x:Class="StepBarControl.MainWindow"
xmlns:stepBar="clr-namespace:StepBarControl"
...>
<Grid>
<stepBar:StepBar Steps="{Binding Steps}" CurrentStep="{Binding CurrentStep}"/>
</Grid>
</Window>
```
3. 在ViewModel中初始化步骤和当前步骤的值:
```csharp
public class MainViewModel : INotifyPropertyChanged
{
private ObservableCollection<string> _steps;
private string _currentStep;
public ObservableCollection<string> Steps
{
get { return _steps; }
set
{
_steps = value;
OnPropertyChanged(nameof(Steps));
}
}
public string CurrentStep
{
get { return _currentStep; }
set
{
_currentStep = value;
OnPropertyChanged(nameof(CurrentStep));
}
}
public MainViewModel()
{
Steps = new ObservableCollection<string>() { "Step 1", "Step 2", "Step 3", "Step 4" };
CurrentStep = "Step 1";
}
}
```
相关推荐
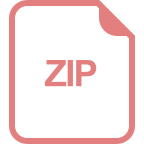
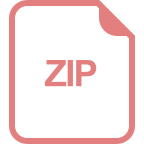
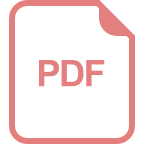














