双流网络的pytorch实现
时间: 2023-07-07 18:34:38 浏览: 186
以下是双流网络的 PyTorch 实现示例:
```python
import torch
import torch.nn as nn
class TwoStreamNet(nn.Module):
def __init__(self, num_classes=10):
super(TwoStreamNet, self).__init__()
# Spatial stream
self.spatial_conv1 = nn.Conv2d(3, 96, kernel_size=7, stride=2, padding=2)
self.spatial_pool1 = nn.MaxPool2d(kernel_size=3, stride=2)
self.spatial_norm1 = nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75)
self.spatial_conv2 = nn.Conv2d(96, 256, kernel_size=5, stride=2, padding=1)
self.spatial_pool2 = nn.MaxPool2d(kernel_size=3, stride=2)
self.spatial_norm2 = nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75)
self.spatial_conv3 = nn.Conv2d(256, 512, kernel_size=3, stride=1, padding=1)
self.spatial_conv4 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.spatial_conv5 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.spatial_pool5 = nn.MaxPool2d(kernel_size=3, stride=2)
# Temporal stream
self.temporal_conv1 = nn.Conv2d(3, 96, kernel_size=7, stride=2, padding=2)
self.temporal_pool1 = nn.MaxPool2d(kernel_size=3, stride=2)
self.temporal_norm1 = nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75)
self.temporal_conv2 = nn.Conv2d(96, 256, kernel_size=5, stride=2, padding=1)
self.temporal_pool2 = nn.MaxPool2d(kernel_size=3, stride=2)
self.temporal_norm2 = nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75)
self.temporal_conv3 = nn.Conv2d(256, 512, kernel_size=3, stride=1, padding=1)
self.temporal_conv4 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.temporal_conv5 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.temporal_pool5 = nn.MaxPool2d(kernel_size=3, stride=2)
# Fully connected layers
self.fc6 = nn.Linear(4096, 4096)
self.dropout6 = nn.Dropout(p=0.5)
self.fc7 = nn.Linear(4096, 4096)
self.dropout7 = nn.Dropout(p=0.5)
self.fc8 = nn.Linear(4096, num_classes)
def forward(self, x):
# Split the input into spatial and temporal streams
spatial = x[:, :, :, 0:224]
temporal = x[:, :, :, 224:448]
# Spatial stream forward
spatial = self.spatial_conv1(spatial)
spatial = self.spatial_pool1(spatial)
spatial = self.spatial_norm1(spatial)
spatial = nn.functional.relu(spatial)
spatial = self.spatial_conv2(spatial)
spatial = self.spatial_pool2(spatial)
spatial = self.spatial_norm2(spatial)
spatial = nn.functional.relu(spatial)
spatial = self.spatial_conv3(spatial)
spatial = nn.functional.relu(spatial)
spatial = self.spatial_conv4(spatial)
spatial = nn.functional.relu(spatial)
spatial = self.spatial_conv5(spatial)
spatial = self.spatial_pool5(spatial)
spatial = nn.functional.relu(spatial)
spatial = spatial.view(spatial.size(0), -1)
# Temporal stream forward
temporal = self.temporal_conv1(temporal)
temporal = self.temporal_pool1(temporal)
temporal = self.temporal_norm1(temporal)
temporal = nn.functional.relu(temporal)
temporal = self.temporal_conv2(temporal)
temporal = self.temporal_pool2(temporal)
temporal = self.temporal_norm2(temporal)
temporal = nn.functional.relu(temporal)
temporal = self.temporal_conv3(temporal)
temporal = nn.functional.relu(temporal)
temporal = self.temporal_conv4(temporal)
temporal = nn.functional.relu(temporal)
temporal = self.temporal_conv5(temporal)
temporal = self.temporal_pool5(temporal)
temporal = nn.functional.relu(temporal)
temporal = temporal.view(temporal.size(0), -1)
# Concatenate the spatial and temporal streams
out = torch.cat([spatial, temporal], dim=1)
# Fully connected layers
out = self.fc6(out)
out = nn.functional.relu(out)
out = self.dropout6(out)
out = self.fc7(out)
out = nn.functional.relu(out)
out = self.dropout7(out)
out = self.fc8(out)
return out
```
这是一个简单的双流网络,其中包含一个空间流和一个时间流,每个流都有自己的卷积和池化层。两个流在最后一个全连接层之前合并。这个模型可以根据需要进行修改和扩展。
阅读全文
相关推荐
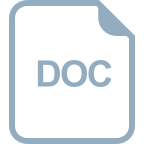
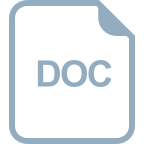
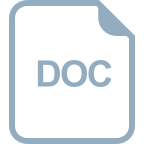
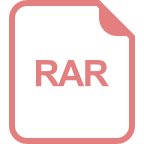
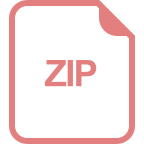
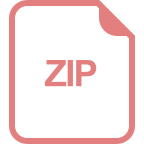
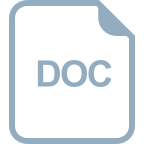
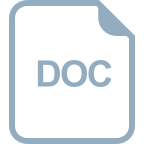
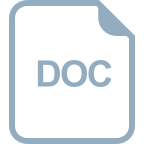
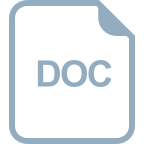
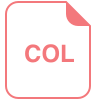
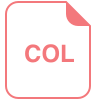
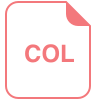


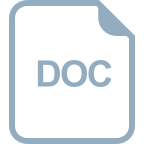
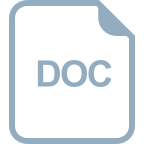
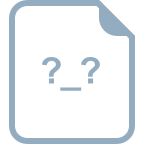
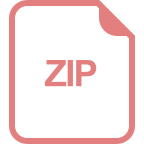
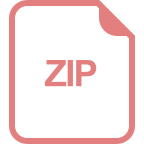