@Overridepublic Course clone() { Course cloned = new Course(courseName); cloned.numberOfStudents = numberOfStudents; cloned.students = Arrays.copyOf(students, students.length); return cloned;}增加一个clone类,实现students的深度复制
时间: 2023-07-14 08:13:39 浏览: 255
可以尝试这样编写 `clone()` 方法:
```
@Override
public Course clone() {
Course cloned = new Course(courseName);
cloned.numberOfStudents = numberOfStudents;
cloned.students = new Student[students.length];
for (int i = 0; i < students.length; i++) {
cloned.students[i] = new Student(students[i].getName(), students[i].getAge());
}
return cloned;
}
```
这里使用了一个 for 循环来遍历原数组中的每个学生对象,并为每个学生对象创建一个新的副本,以实现深度复制。需要注意的是,这里假设 `Student` 类有一个带参数的构造方法,用于传递学生的姓名和年龄信息。如果 `Student` 类没有这个构造方法,需要根据实际情况进行修改。
相关问题
John is working in Software Company as a developer, he has developed a web application following client-server architecture and he wants to implement Spring REST API on client application for getting the list of the studentsl from the database. Which of the following code snippet he will use to get the desired result?@Overridepublic List<Student> getStudent() {ResponseEntity<List<Student>> responseEntity =restTemplate.exchange(studentRestUrl, HttpMethod.GET,new ParameterizedTypeReference<List<Student>>() {});AList<Student> students = responseEntity.getBody();} return students;
The code snippet that John will use to get the list of students from the database using Spring REST API on the client application would be:
```
@Override
public List<Student> getStudent() {
ResponseEntity<List<Student>> responseEntity = restTemplate.exchange(
studentRestUrl,
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<Student>>() {}
);
List<Student> students = responseEntity.getBody();
return students;
}
```
In this code, John is using the Spring RestTemplate to send an HTTP GET request to the server-side REST API endpoint at the `studentRestUrl`. The `exchange()` method is used to send the request and receive the response, with the HTTP method type as `GET` and the `ParameterizedTypeReference` specifying the expected response type as a list of `Student` objects. The response is then extracted using the `getBody()` method of the `ResponseEntity` object and returned as a list of `Student` objects.
private JButton addButton;public InterfaceCallExample() { //... addButton = new JButton("新增数据"); addButton.addActionListener(this); panel.add(addButton); //...}@Overridepublic void actionPerformed(ActionEvent e) { if (e.getSource() == callButton) { //... } else if (e.getSource() == addButton) { String id = JOptionPane.showInputDialog(this, "请输入id:"); String titleName = JOptionPane.showInputDialog(this, "请输入材料类型:"); String status = JOptionPane.showInputDialog(this, "请输入状态:"); String createTime = JOptionPane.showInputDialog(this, "请输入创建时间:"); String updateTime = JOptionPane.showInputDialog(this, "请输入更改时间:"); ArrayList<String> strings = new ArrayList<>(); strings.add(id); strings.add(titleName); strings.add(status); strings.add(createTime); strings.add(updateTime); String[] data = strings.toString().replace("[","").replace("]","").split(","); tableModel.addRow(data); }} 把新增的输入框可以一次性展示出来
您可以将输入框放在一个对话框中,以便一次性展示出来。可以使用以下代码:
```java
else if (e.getSource() == addButton) {
JPanel panel = new JPanel(new GridLayout(0, 1));
JTextField idField = new JTextField(10);
JTextField titleNameField = new JTextField(10);
JTextField statusField = new JTextField(10);
JTextField createTimeField = new JTextField(10);
JTextField updateTimeField = new JTextField(10);
panel.add(new JLabel("id:"));
panel.add(idField);
panel.add(new JLabel("材料类型:"));
panel.add(titleNameField);
panel.add(new JLabel("状态:"));
panel.add(statusField);
panel.add(new JLabel("创建时间:"));
panel.add(createTimeField);
panel.add(new JLabel("更改时间:"));
panel.add(updateTimeField);
int result = JOptionPane.showConfirmDialog(null, panel, "新增数据", JOptionPane.OK_CANCEL_OPTION, JOptionPane.PLAIN_MESSAGE);
if (result == JOptionPane.OK_OPTION) {
String id = idField.getText();
String titleName = titleNameField.getText();
String status = statusField.getText();
String createTime = createTimeField.getText();
String updateTime = updateTimeField.getText();
ArrayList<String> strings = new ArrayList<>();
strings.add(id);
strings.add(titleName);
strings.add(status);
strings.add(createTime);
strings.add(updateTime);
String[] data = strings.toString().replace("[","").replace("]","").split(",");
tableModel.addRow(data);
}
}
```
这段代码将会弹出一个带有多个输入框的对话框,用户可以在其中输入数据,单击“确定”按钮后,将会向表格中添加一行数据。
阅读全文
相关推荐
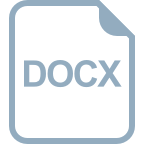
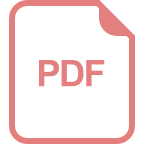
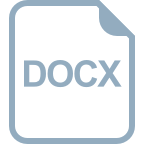






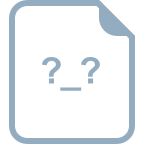
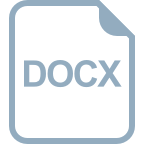
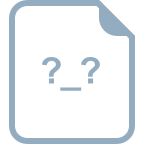
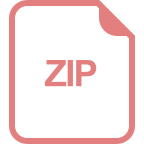
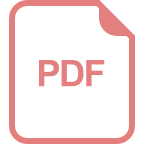
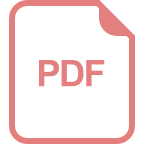
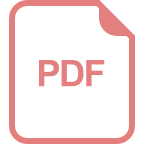
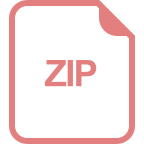
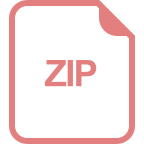