用Java 函数的参数和返回值。(自定义一个计算器,输入几个头,几个爪,几碗面,单价自己定,并且计算总价)(使用四种方式来完成)
时间: 2023-12-07 13:06:06 浏览: 33
1. 使用基本数据类型实现:
```java
public class Calculator {
public static void main(String[] args) {
int head = 2;
int claw = 4;
int bowl = 3;
double price = 12.5;
double total = calculateTotal(head, claw, bowl, price);
System.out.println("总价为:" + total);
}
public static double calculateTotal(int head, int claw, int bowl, double price) {
return (head * 2 + claw * 3 + bowl * 1.5) * price;
}
}
```
2. 使用数组实现:
```java
public class Calculator {
public static void main(String[] args) {
int[] nums = new int[]{2, 4, 3};
double price = 12.5;
double total = calculateTotal(nums, price);
System.out.println("总价为:" + total);
}
public static double calculateTotal(int[] nums, double price) {
return (nums[0] * 2 + nums[1] * 3 + nums[2] * 1.5) * price;
}
}
```
3. 使用对象实现:
```java
public class Calculator {
public static void main(String[] args) {
Goods goods = new Goods(2, 4, 3, 12.5);
double total = calculateTotal(goods);
System.out.println("总价为:" + total);
}
public static double calculateTotal(Goods goods) {
return (goods.getHead() * 2 + goods.getClaw() * 3 + goods.getBowl() * 1.5) * goods.getPrice();
}
}
class Goods {
private int head;
private int claw;
private int bowl;
private double price;
public Goods(int head, int claw, int bowl, double price) {
this.head = head;
this.claw = claw;
this.bowl = bowl;
this.price = price;
}
public int getHead() {
return head;
}
public void setHead(int head) {
this.head = head;
}
public int getClaw() {
return claw;
}
public void setClaw(int claw) {
this.claw = claw;
}
public int getBowl() {
return bowl;
}
public void setBowl(int bowl) {
this.bowl = bowl;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
4. 使用Map实现:
```java
import java.util.HashMap;
import java.util.Map;
public class Calculator {
public static void main(String[] args) {
Map<String, Integer> goods = new HashMap<>();
goods.put("head", 2);
goods.put("claw", 4);
goods.put("bowl", 3);
double price = 12.5;
double total = calculateTotal(goods, price);
System.out.println("总价为:" + total);
}
public static double calculateTotal(Map<String, Integer> goods, double price) {
int head = goods.get("head");
int claw = goods.get("claw");
int bowl = goods.get("bowl");
return (head * 2 + claw * 3 + bowl * 1.5) * price;
}
}
```
相关推荐
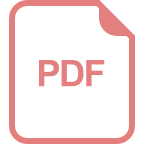
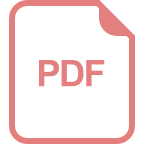














