yolov5识别项目打包
时间: 2023-10-14 09:05:54 浏览: 65
您可以使用Python的PyInstaller库将YOLOv5项目打包成可执行文件。以下是一些步骤供您参考:
1. 首先,确保您的YOLOv5项目已经配置好并可以成功运行。
2. 安装PyInstaller库。您可以使用以下命令在终端或命令提示符中安装:
```
pip install pyinstaller
```
3. 进入您的YOLOv5项目的根目录。
4. 使用PyInstaller将项目打包为可执行文件。运行以下命令:
```
pyinstaller --onefile your_script.py
```
其中 "your_script.py" 是包含您的YOLOv5识别代码的Python脚本文件名。
5. 打包过程完成后,在生成的 "dist" 文件夹中会有一个可执行文件。
6. 您可以将生成的可执行文件复制到其他计算机上运行,而无需安装Python环境或任何依赖项。
相关问题
yolov5识别水坑
yolov5是一种先进的目标检测算法,可以识别图像中的各种物体。要让yolov5识别水坑,首先需要准备大量的包含水坑的图像数据,包括不同角度、不同光照条件下的水坑图片。然后利用这些图像数据进行训练,让yolov5学习如何识别水坑。在训练的过程中,需要不断调整算法的参数,使之更加适应水坑的特征。
在训练完成后,可以使用训练好的yolov5模型来识别图像中的水坑。将待识别的图像输入到yolov5模型中,模型会自动判断图像中是否存在水坑,并给出识别结果。同时,可以通过对yolov5模型进行微调,进一步提高水坑的识别准确度和鲁棒性。
通过yolov5识别水坑,可以应用于诸如环境监测、路况监测等领域,帮助人们更好地了解周围环境并做出相应的决策。同时,还可以为水坑的自动驾驶汽车、无人机等技术提供重要的支持,提高其在复杂环境中的应用能力。总之,yolov5识别水坑是一个具有广泛应用前景的技术,将为人们的生活和工作带来诸多便利。
yolov5识别植物
YOLOv5是一种目标检测算法,它可以用于识别图像或视频中的各种物体,包括植物。下面是YOLOv5识别植物的一般步骤:
1. 数据准备:收集包含植物的图像数据集,并进行标注,标注每个图像中的植物位置和类别。
2. 模型训练:使用YOLOv5的训练脚本,将准备好的数据集输入到模型中进行训练。在训练过程中,模型会学习如何检测和分类植物。
3. 模型评估:使用测试数据集对训练好的模型进行评估,计算模型的准确率、召回率等指标,以评估模型的性能。
4. 模型应用:将训练好的模型应用于新的图像或视频中,通过模型的推理过程,可以检测和识别图像中的植物,并输出它们的位置和类别。
相关问题:
1. YOLOv5是什么?
2. YOLOv5如何进行目标检测?
3. YOLOv5需要哪些数据来进行训练?
4. YOLOv5在植物识别方面有哪些应用场景?
相关推荐
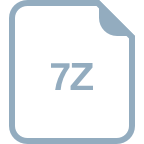
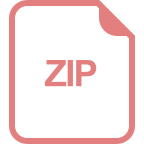
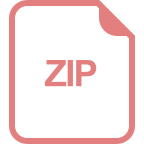












