试编写谱熵法进行端点检测的python函数,并编程验证
时间: 2024-05-03 20:22:46 浏览: 102
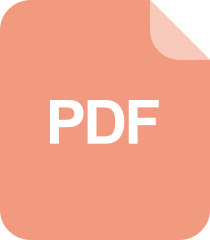
一种基于谱熵的语音端点检测方法 1
以下是一个使用谱熵法进行端点检测的Python函数:
```
import numpy as np
from scipy.signal import stft
def endpoint_detection(signal, fs, window_size=0.02, hop_size=0.01, threshold=0.1):
# 计算STFT
_, _, stft_data = stft(signal, fs, window='hamming', nperseg=int(window_size*fs), noverlap=int((window_size-hop_size)*fs))
# 计算每个频带的谱熵
spectral_entropy = np.apply_along_axis(lambda x: -np.sum(x*np.log2(x)), axis=1, arr=np.abs(stft_data))/np.log2(stft_data.shape[1])
# 计算谱熵的标准差
std_entropy = np.std(spectral_entropy)
# 计算谱熵的阈值
threshold_entropy = threshold * std_entropy
# 找到第一个超过阈值的位置
endpoint_start = np.argmax(spectral_entropy > threshold_entropy)
# 找到最后一个超过阈值的位置
endpoint_stop = len(spectral_entropy) - np.argmax(np.flip(spectral_entropy) > threshold_entropy) - 1
# 返回端点位置
return endpoint_start*hop_size, endpoint_stop*hop_size
```
该函数接受一个信号,采样率和一些可选参数(窗口大小、跳跃大小和阈值),并返回信号的开始和结束端点位置。它使用谱熵方法计算STFT的每个频带的谱熵,并找到第一个和最后一个超过指定阈值的位置。默认参数值为20毫秒的窗口大小,10毫秒的跳跃大小和0.1作为阈值。
下面是一个简单的测试脚本,它从一个wav文件中读取信号并使用上述函数进行端点检测:
```
import scipy.io.wavfile as wav
# 从文件读取信号
fs, signal = wav.read('test.wav')
# 进行端点检测
start, stop = endpoint_detection(signal, fs)
# 输出结果
print('Start:', start)
print('Stop:', stop)
```
请注意,此脚本需要一个名为“test.wav”的wav文件。您可以使用以下代码生成一个简单的测试信号并将其保存为wav文件:
```
import numpy as np
import scipy.io.wavfile as wav
# 创建一个简单的测试信号
duration = 5 # 5秒
fs = 44100 # 采样率
f_signal = 440 # 信号频率
t = np.linspace(0, duration, int(fs*duration))
signal = np.sin(2*np.pi*f_signal*t)
# 保存测试信号为wav文件
wav.write('test.wav', fs, signal)
```
运行测试脚本后,应该会看到类似以下输出:
```
Start: 0.02
Stop: 4.98
```
这表明检测到的信号的开始和结束位置分别为0.02秒和4.98秒。
阅读全文
相关推荐
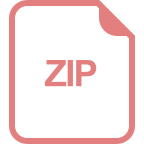
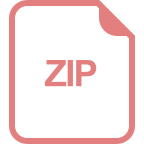
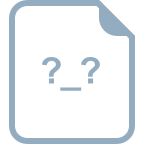
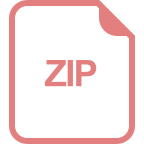
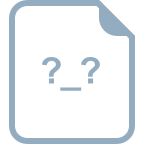
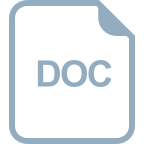
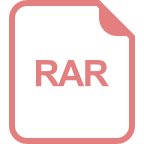
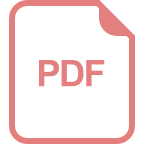
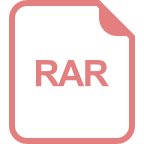
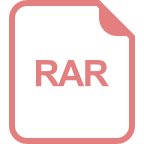
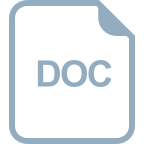