springboot controller 异步大文件下载
时间: 2023-07-26 19:18:42 浏览: 118
要实现Spring Boot中Controller异步大文件下载,可以使用Servlet 3.0中的异步特性。
首先,在Controller中定义一个异步方法,使用HttpServletResponse将文件流写回客户端。具体代码如下:
```java
@GetMapping("/download")
public void download(HttpServletResponse response) throws Exception {
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=file.zip");
AsyncContext asyncContext = request.startAsync();
asyncContext.setTimeout(0);
asyncContext.start(() -> {
try {
OutputStream outputStream = response.getOutputStream();
InputStream inputStream = new FileInputStream(new File("path/to/large/file"));
IOUtils.copy(inputStream, outputStream);
outputStream.flush();
asyncContext.complete();
} catch (Exception e) {
asyncContext.complete();
throw new RuntimeException(e);
}
});
}
```
在上面的代码中,我们使用AsyncContext启动一个异步线程,将文件流写回客户端。同时,我们需要设置异步超时时间为0,以避免Servlet容器默认的超时时间限制。
另外,我们使用了Apache Commons IO库中的IOUtils.copy方法,将输入流复制到输出流中。
最后,需要注意的是,由于文件下载是一个比较耗时的操作,因此建议将下载请求交给专门的线程池进行处理,以避免阻塞其他请求。可以使用Spring Boot提供的@Async注解将方法标记为异步方法,并配置线程池参数。
```java
@Configuration
@EnableAsync
public class AsyncConfig implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(100);
executor.setThreadNamePrefix("async-download-");
executor.initialize();
return executor;
}
}
```
在上面的代码中,我们配置了一个线程池,核心线程数为10,最大线程数为20,队列容量为100,线程名前缀为async-download-。这样,每个下载请求都会由一个线程处理,避免了阻塞其他请求的情况。
阅读全文
相关推荐
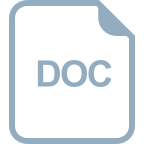
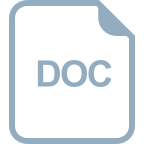
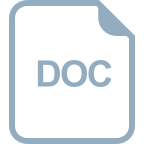

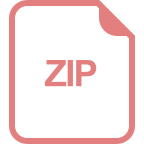
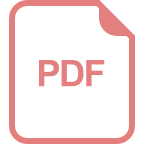
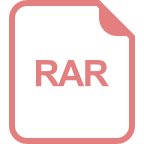
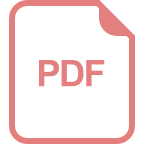
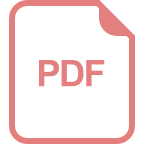
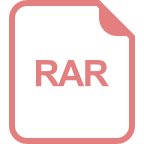
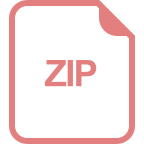
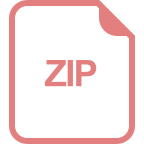
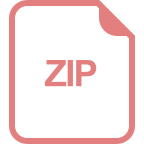
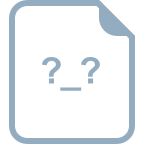
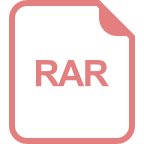
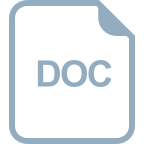
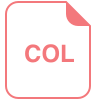
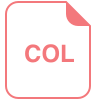
