利用Promise和async/await实现以下功能: 现有一个接口https://test.com/get?id=1,需要发起id从1~200的GET方式调用200次异步请求,要求分20轮发出,前一轮全部完成后才进行下一轮,每轮同时并发请求为10次。
时间: 2024-01-15 20:03:59 浏览: 26
利用Promise和async/await实现如下:
```javascript
const fetchData = async (id) => {
const url = `https://test.com/get?id=${id}`;
const response = await fetch(url);
const data = await response.json();
return data;
};
const fetchAllData = async () => {
const roundCount = 20;
const concurrencyCount = 10;
const idCount = 200;
for (let i = 0; i < roundCount; i++) {
const roundStartId = i * concurrencyCount + 1;
const roundEndId = (i + 1) * concurrencyCount;
const promises = [];
for (let j = roundStartId; j <= roundEndId && j <= idCount; j++) {
promises.push(fetchData(j));
}
await Promise.all(promises);
}
};
fetchAllData();
```
首先,定义了一个`fetchData`函数,用于发起单次异步请求并返回数据。
然后,定义了一个`fetchAllData`函数,用于发起200次异步请求。该函数中有3个重要的变量:
- `roundCount` 表示总共需要发起的轮数,即20轮;
- `concurrencyCount` 表示每轮同时并发的请求数量,即10次;
- `idCount` 表示总共需要请求的id数,即200个。
接着,使用一个`for`循环,依次发起每一轮的请求。在每一轮中,先计算出该轮的起始id和结束id,然后构建一个Promise数组,用于存储该轮所有的异步请求。在内层的`for`循环中,依次发起每个异步请求,并将其Promise对象存储到数组中。最后,使用`Promise.all`方法等待该轮所有异步请求完成后,再进行下一轮的请求。
相关推荐
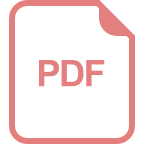
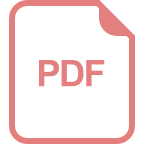











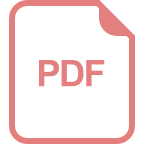
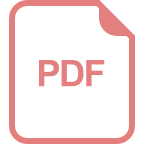
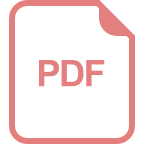