管理员对用户发布的信息具有审批和撤销功能,ssm框架怎么实现代码,从设计数据库开始一步步实现,以及前端代码
时间: 2023-12-06 09:44:17 浏览: 28
1. 设计数据库
首先需要设计数据库,假设有两张表:用户信息表和用户发布信息表。
用户信息表包括以下字段:
| 字段名 | 类型 | 描述 |
| ---------- | -------- | -------- |
| user_id | int | 用户ID |
| user_name | varchar | 用户名 |
| user_pass | varchar | 用户密码 |
| user_level | int | 用户等级 |
用户发布信息表包括以下字段:
| 字段名 | 类型 | 描述 |
| -------------- | --------- | ---------------- |
| info_id | int | 信息ID |
| user_id | int | 用户ID |
| info_title | varchar | 信息标题 |
| info_content | varchar | 信息内容 |
| info_time | datetime | 信息发布时间 |
| info_is_passed | tinyint | 信息是否已审批 |
| info_is_delete | tinyint | 信息是否已删除 |
2. 编写后端代码
使用SSM框架编写后端代码,具体步骤如下:
1.创建User和Info的实体类
```
public class User {
private int user_id;
private String user_name;
private String user_pass;
private int user_level;
//省略getter和setter方法
}
public class Info {
private int info_id;
private int user_id;
private String info_title;
private String info_content;
private Date info_time;
private int info_is_passed;
private int info_is_delete;
//省略getter和setter方法
}
```
2.创建User和Info的Mapper接口
```
public interface UserMapper {
User selectUserById(int user_id);
}
public interface InfoMapper {
List<Info> selectAllInfoByUserId(int user_id);
Info selectInfoById(int info_id);
void insertInfo(Info info);
void updateInfo(Info info);
}
```
3.创建User和Info的Service接口和实现类
```
public interface UserService {
User getUserById(int user_id);
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(int user_id) {
return userMapper.selectUserById(user_id);
}
}
public interface InfoService {
List<Info> getAllInfoByUserId(int user_id);
Info getInfoById(int info_id);
void addInfo(Info info);
void updateInfo(Info info);
}
@Service
public class InfoServiceImpl implements InfoService {
@Autowired
private InfoMapper infoMapper;
@Override
public List<Info> getAllInfoByUserId(int user_id) {
return infoMapper.selectAllInfoByUserId(user_id);
}
@Override
public Info getInfoById(int info_id) {
return infoMapper.selectInfoById(info_id);
}
@Override
public void addInfo(Info info) {
infoMapper.insertInfo(info);
}
@Override
public void updateInfo(Info info) {
infoMapper.updateInfo(info);
}
}
```
4.创建Controller类
```
@Controller
@RequestMapping("/info")
public class InfoController {
@Autowired
private UserService userService;
@Autowired
private InfoService infoService;
@RequestMapping("/list")
public String list(Model model, HttpSession session) {
int user_id = (int) session.getAttribute("user_id");
List<Info> infoList = infoService.getAllInfoByUserId(user_id);
model.addAttribute("infoList", infoList);
return "info_list";
}
@RequestMapping("/add")
public String add(Model model) {
model.addAttribute("info", new Info());
return "info_add";
}
@RequestMapping("/save")
public String save(@ModelAttribute("info") Info info, HttpSession session) {
int user_id = (int) session.getAttribute("user_id");
info.setUser_id(user_id);
info.setInfo_time(new Date());
info.setInfo_is_passed(0); // 初始状态为未审批
info.setInfo_is_delete(0); // 初始状态为未删除
infoService.addInfo(info);
return "redirect:/info/list";
}
@RequestMapping("/edit")
public String edit(@RequestParam("info_id") int info_id, Model model) {
Info info = infoService.getInfoById(info_id);
model.addAttribute("info", info);
return "info_edit";
}
@RequestMapping("/update")
public String update(@ModelAttribute("info") Info info) {
infoService.updateInfo(info);
return "redirect:/info/list";
}
}
```
3. 编写前端代码
使用HTML和Thymeleaf模板引擎编写前端代码,具体步骤如下:
1.创建信息列表页面(info_list.html)
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>信息列表</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>标题</th>
<th>内容</th>
<th>发布时间</th>
<th>审批状态</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="info : ${infoList}">
<td th:text="${info.info_id}"></td>
<td th:text="${info.info_title}"></td>
<td th:text="${info.info_content}"></td>
<td th:text="${info.info_time}"></td>
<td th:text="${info.info_is_passed == 0 ? '未审批' : '已审批'}"></td>
<td>
<a th:href="@{/info/edit(info_id=${info.info_id})}">编辑</a>
</td>
</tr>
</tbody>
</table>
<a th:href="@{/info/add}">添加信息</a>
</body>
</html>
```
2.创建添加信息页面(info_add.html)
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>添加信息</title>
</head>
<body>
<form th:action="@{/info/save}" th:object="${info}" method="post">
<label>标题:</label>
<input type="text" th:field="*{info_title}"><br>
<label>内容:</label>
<textarea th:field="*{info_content}"></textarea><br>
<input type="submit" value="保存">
</form>
</body>
</html>
```
3.创建编辑信息页面(info_edit.html)
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>编辑信息</title>
</head>
<body>
<form th:action="@{/info/update}" th:object="${info}" method="post">
<input type="hidden" th:field="*{info_id}">
<label>标题:</label>
<input type="text" th:field="*{info_title}"><br>
<label>内容:</label>
<textarea th:field="*{info_content}"></textarea><br>
<input type="submit" value="保存">
</form>
</body>
</html>
```
至此,使用SSM框架实现管理员对用户发布的信息具有审批和撤销功能的代码就完成了。
相关推荐
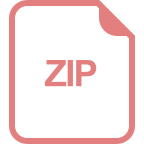
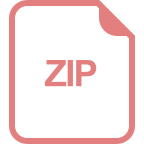
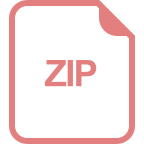
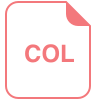
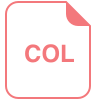
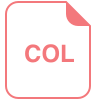
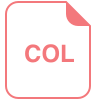
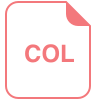









