定义一个表示学生的student类,包括的域有学号、姓名、性别、年龄,身高,体重;编写学生类的无参构造方法。编写所有属性的getters和setters方法。为上题的student类定义构造函数初始化
时间: 2023-05-31 11:19:45 浏览: 93
### 回答1:
学生类的定义如下:
```
public class Student {
private String id; // 学号
private String name; // 姓名
private String gender; // 性别
private int age; // 年龄
private double height; // 身高
private double weight; // 体重
// 无参构造方法
public Student() {}
// 构造函数初始化
public Student(String id, String name, String gender, int age, double height, double weight) {
this.id = id;
this.name = name;
this.gender = gender;
this.age = age;
this.height = height;
this.weight = weight;
}
// getters和setters方法
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
```
### 回答2:
学生是我们生活中经常接触到的人群,他们是我们国家未来的希望,也是我们的朋友。下面我将为大家定义一个表示学生的Studnet类,包括的域有学号、姓名、性别、年龄,身高和体重,并编写学生类的无参构造方法,以及所有属性的getters和setters方法。同时,也为该Student类定义了构造函数实现属性的初始化。
Student类的定义:
public class Student {
private String id; // 学号
private String name; // 姓名
private String sex; // 性别
private int age; // 年龄
private double height; // 身高
private double weight; // 体重
// 学生类的无参构造方法
public Student(){}
// 为所有属性定义getters和setters方法
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
// 为Student类定义构造函数初始化属性
public Student(String id, String name, String sex, int age, double height, double weight) {
this.id = id;
this.name = name;
this.sex = sex;
this.age = age;
this.height = height;
this.weight = weight;
}
}
以上就是一个简单的表示学生的Student类,它包含了具体的属性和方法,便于我们在程序中使用。可以使用无参构造方法来创建该类的实例对象,并通过getters和setters方法来操作该实例。同时,也可以使用构造函数进行属性的初始化。
### 回答3:
学生是教育系统中的一个重要组成部分,因此需要定义一个表示学生的类。该类需要包括学号、姓名、性别、年龄、身高和体重等基本属性。此外,还需要为该类编写无参构造方法和getters和setters方法,以便我们可以方便地访问和操作这些属性。
首先,我们可以定义一个名为Student的类来表示学生。该类的属性包括学号、姓名、性别、年龄、身高和体重,如下所示:
public class Student {
private String studentId;
private String name;
private String gender;
private int age;
private double height;
private double weight;
}
接下来,我们可以为该类编写无参构造方法和getters和setters方法:
public Student() {
// 无参构造方法
}
public String getStudentId() {
return studentId;
}
public void setStudentId(String studentId) {
this.studentId = studentId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
最后,我们为该类添加一个带参数的构造函数,以便我们可以在创建对象时初始化该类的所有属性:
public Student(String studentId, String name, String gender, int age, double height, double weight) {
this.studentId = studentId;
this.name = name;
this.gender = gender;
this.age = age;
this.height = height;
this.weight = weight;
}
通过以上这些基本操作,我们可以方便地创建、初始化和修改学生对象的属性。同时,该类还可以扩展其他功能,如计算BMI指数等。
相关推荐
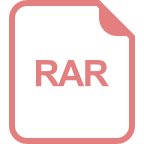
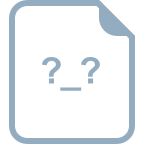
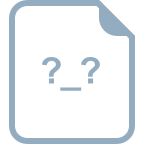














