yolov7摄像头显示实时人数
时间: 2023-06-27 15:08:34 浏览: 146
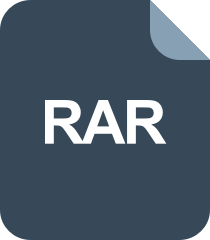
正原基于STM32F1的ov7725摄像头,实时显示

要实现YOLOv7模型实时检测摄像头中的人数,你可以按照以下步骤进行操作:
1. 安装所需的库:opencv-python, torch, torchvision, numpy
2. 下载YOLOv7模型的权重文件,可以从GitHub上下载 https://github.com/WongKinYiu/yolov7 。
3. 编写Python脚本,使用OpenCV读取摄像头的视频流,然后使用YOLOv7模型对每帧图像进行目标检测。在检测到人的情况下,记录人数并将其显示在视频流上。
以下是一个简单的示例代码:
```python
import cv2
import torch
import numpy as np
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords, xyxy2xywh
# 加载YOLOv7模型
weights = 'yolov7.pt'
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max())
imgsz = int(640 / stride * stride + stride)
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头视频流
ret, frame = cap.read()
# 图像预处理
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (imgsz, imgsz))
img = torch.from_numpy(img).float().to(device)
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 使用YOLOv7模型进行目标检测
pred = model(img)[0]
pred = non_max_suppression(pred, 0.4, 0.5)
# 统计检测到的人数
num_people = 0
for det in pred:
if det is not None and len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
for *xyxy, conf, cls in reversed(det):
if int(cls) == 0: # 0代表person类别
num_people += 1
label = f'Person {num_people}'
plot_one_box(xyxy, frame, label=label, color=(0, 255, 0), line_thickness=3)
# 在视频流上显示当前检测到的人数
cv2.putText(frame, f'People: {num_people}', (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
cv2.imshow('Camera', frame)
# 按'q'键退出程序
key = cv2.waitKey(1)
if key == ord('q'):
break
# 释放摄像头并关闭所有窗口
cap.release()
cv2.destroyAllWindows()
```
请注意,此代码仅供参考,需要根据实际情况进行修改。例如,您可能需要调整目标检测的阈值、颜色等参数以适应不同的场景。
阅读全文
相关推荐
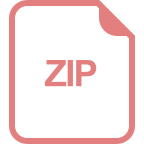
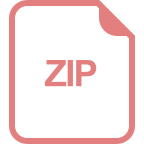
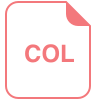
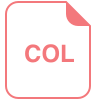

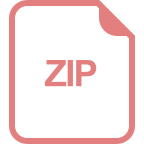
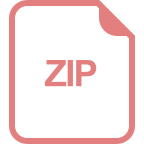
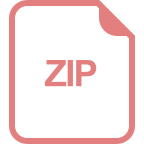