pyqt5编写yolov8可视化界面
时间: 2023-10-01 15:08:34 浏览: 433
PyQt5 是一个基于 Python 的 GUI 编程库,可以用来创建各种各样的桌面应用程序。YOLOv8 则是一种目标检测算法的实现,可以用于检测图像或视频中的物体。
要编写 YOLOv8 的可视化界面,你需要掌握以下技能:
1. PyQt5 的基本使用:包括窗口、布局、控件等的创建和使用。
2. YOLOv8 的基本使用:包括数据集的准备、模型的训练和测试等。
3. 图像处理的基本知识:包括图像的读取、显示、缩放等操作。
下面是一个简单的示例,演示如何使用 PyQt5 创建一个简单的 YOLOv8 可视化界面:
```python
import sys
import cv2
import numpy as np
from PyQt5.QtCore import Qt, QTimer
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QDialog, QLabel, QVBoxLayout, QHBoxLayout, QPushButton, QFileDialog
from yolo import YOLOv8
class App(QDialog):
def __init__(self):
super().__init__()
# 创建 YOLOv8 模型
self.yolo = YOLOv8()
# 创建 UI 控件
self.image_label = QLabel(self)
self.image_label.setAlignment(Qt.AlignCenter)
self.image_label.setMinimumSize(640, 480)
self.start_button = QPushButton('Start', self)
self.start_button.clicked.connect(self.start)
self.stop_button = QPushButton('Stop', self)
self.stop_button.clicked.connect(self.stop)
self.stop_button.setEnabled(False)
self.open_button = QPushButton('Open', self)
self.open_button.clicked.connect(self.open)
# 创建布局
buttons_layout = QHBoxLayout()
buttons_layout.addWidget(self.start_button)
buttons_layout.addWidget(self.stop_button)
buttons_layout.addWidget(self.open_button)
main_layout = QVBoxLayout()
main_layout.addWidget(self.image_label)
main_layout.addLayout(buttons_layout)
self.setLayout(main_layout)
# 创建计时器
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_frame)
# 初始化状态
self.cap = None
self.started = False
def start(self):
# 打开摄像头
self.cap = cv2.VideoCapture(0)
# 启动计时器
self.timer.start(33)
# 更新 UI 控件
self.start_button.setEnabled(False)
self.stop_button.setEnabled(True)
self.open_button.setEnabled(False)
self.started = True
def stop(self):
# 停止计时器
self.timer.stop()
# 关闭摄像头
self.cap.release()
# 更新 UI 控件
self.start_button.setEnabled(True)
self.stop_button.setEnabled(False)
self.open_button.setEnabled(True)
self.started = False
def open(self):
# 打开文件对话框
file_name, _ = QFileDialog.getOpenFileName(self, 'Open File', '', 'Images (*.png *.xpm *.jpg *.bmp);;All Files (*.*)')
if file_name:
# 加载图像
self.cap = cv2.VideoCapture(file_name)
# 启动计时器
self.timer.start(33)
# 更新 UI 控件
self.start_button.setEnabled(False)
self.stop_button.setEnabled(True)
self.open_button.setEnabled(False)
self.started = True
def update_frame(self):
# 读取图像
ret, frame = self.cap.read()
if ret:
# 将图像转为 RGB 格式
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 缩放图像
frame = cv2.resize(frame, (640, 480))
# 检测目标
result = self.yolo.detect(frame)
# 绘制边框和标签
for label, x1, y1, x2, y2 in result:
cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(frame, label, (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 将图像转为 QImage 格式
image = QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888)
# 在 UI 控件中显示图像
self.image_label.setPixmap(QPixmap.fromImage(image))
else:
# 如果读取到了最后一帧,停止计时器
self.timer.stop()
# 关闭摄像头
self.cap.release()
# 更新 UI 控件
self.start_button.setEnabled(True)
self.stop_button.setEnabled(False)
self.open_button.setEnabled(True)
self.started = False
if __name__ == '__main__':
# 创建应用程序
app = QApplication(sys.argv)
# 创建主窗口
window = App()
window.setWindowTitle('YOLOv8 GUI')
# 显示窗口
window.show()
# 运行应用程序
sys.exit(app.exec_())
```
这个示例中,我们使用 PyQt5 创建了一个包含三个按钮和一个标签的窗口。当用户单击 “Open” 按钮时,会打开一个文件对话框,用户可以选择要加载的图像文件。单击 “Start” 按钮后,程序会打开摄像头,并在标签中显示摄像头捕获的图像。单击 “Stop” 按钮则会停止显示图像。在显示图像时,程序还会使用 YOLOv8 模型检测图像中的目标,并在图像上绘制边框和标签。
这只是一个简单的示例,你可以根据自己的需求进行修改和扩展。
相关推荐
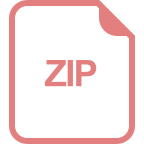
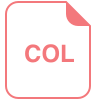
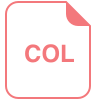
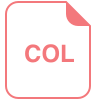
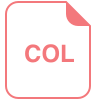
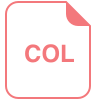









