请写出完整的python代码,实现利用ylov5对包含任意数量香蕉的照片进行计数,并且利用pyqt设计一个界面,实现从文件夹选择图像,显示所选择的图像,点击按钮开始计数,输出计数结果和结果图像四个功能
时间: 2024-04-11 18:30:00 浏览: 107
以下是实现您描述的功能的Python代码,使用了yolov5和PyQt库:
```python
import sys
import os
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QPushButton, QFileDialog
from PyQt5.QtGui import QPixmap
import torch
import cv2
from PIL import Image
from yolov5 import detect
class BananaCounter(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.setWindowTitle("Banana Counter")
self.setGeometry(100, 100, 400, 300)
self.image_label = QLabel(self)
self.result_label = QLabel(self)
self.result_label.setGeometry(10, 200, 380, 50)
self.result_label.setStyleSheet("font-size: 16px; color: red")
self.select_button = QPushButton("Select Image", self)
self.select_button.setGeometry(10, 10, 120, 30)
self.select_button.clicked.connect(self.select_image)
self.count_button = QPushButton("Start Counting", self)
self.count_button.setGeometry(150, 10, 120, 30)
self.count_button.clicked.connect(self.count_bananas)
self.layout = QVBoxLayout()
self.layout.addWidget(self.image_label)
self.layout.addWidget(self.result_label)
self.layout.addWidget(self.select_button)
self.layout.addWidget(self.count_button)
self.setLayout(self.layout)
def select_image(self):
file_dialog = QFileDialog()
image_path, _ = file_dialog.getOpenFileName(self, "Select Image", filter="Image Files (*.png *.jpg)")
if image_path:
self.show_selected_image(image_path)
def show_selected_image(self, image_path):
pixmap = QPixmap(image_path)
self.image_label.setPixmap(pixmap.scaled(380, 180))
def count_bananas(self):
image_path = self.get_selected_image_path()
if image_path:
result_image_path, count = self.detect_bananas(image_path)
self.show_result(count)
self.show_result_image(result_image_path)
def get_selected_image_path(self):
if self.image_label.pixmap():
return self.image_label.pixmap().toImage().text()
return None
def detect_bananas(self, image_path):
weights = 'path/to/your/yolov5/weights.pt'
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# Load model
model = torch.hub.load('ultralytics/yolov5', 'custom', path=weights)
model.to(device).eval()
# Load image
img = Image.open(image_path)
# Inference
results = model(img)
# Get result image path
result_image_path = os.path.splitext(image_path)[0] + '_result.jpg'
results.save(result_image_path)
# Count bananas
count = len(results.pred)
return result_image_path, count
def show_result(self, count):
self.result_label.setText(f"Banana Count: {count}")
def show_result_image(self, result_image_path):
pixmap = QPixmap(result_image_path)
self.result_label.setPixmap(pixmap.scaled(380, 50))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = BananaCounter()
window.show()
sys.exit(app.exec_())
```
请将 `path/to/your/yolov5/weights.pt` 替换为实际的yolov5权重文件路径。另外,您需要在您的环境中安装PyQt和yolov5库,可以使用以下命令进行安装:
```shell
pip install PyQt5 torch torchvision opencv-python Pillow
pip install git+https://github.com/ultralytics/yolov5.git
```
请确保您的环境已安装正确的依赖项。运行代码后,将会弹出一个界面,您可以选择图像文件,点击“Start Counting”按钮进行香蕉计数,并在界面上显示计数结果和结果图像。
阅读全文
相关推荐
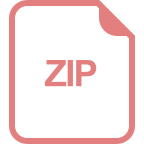
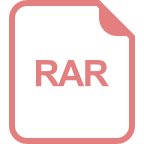
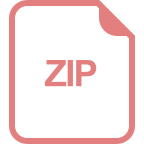
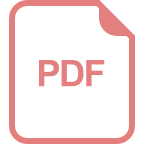
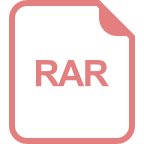
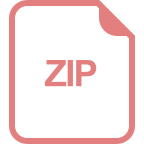
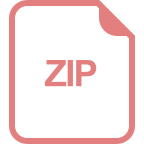
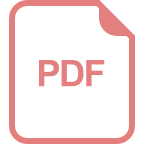
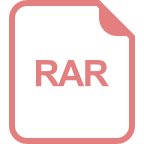
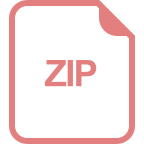
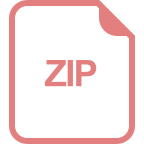
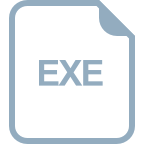
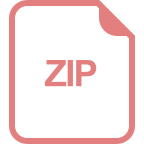
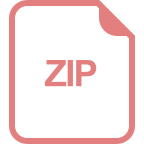
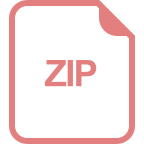
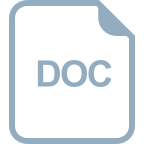