import numpy as np import random # 定义能量函数 def calculate_energy(weights): # 计算投资组合的风险和收益 # 根据权重计算投资组合的收益 returns = np.dot(mu, weights) # 根据权重计算投资组合的风险(标准差) risk = np.sqrt(np.dot(weights.T, np.dot(S, weights))) return risk, returns # 模拟退火算法参数 INITIAL_TEMPERATURE = 100.0 FINAL_TEMPERATURE = 0.1 NUM_ITERATIONS = 1000 # 初始化权重 current_weights = np.ones(len(mu)) / len(mu) # 初始化能量和最优解 current_risk, current_returns = calculate_energy(current_weights) best_risk, best_returns = current_risk, current_returns best_weights = current_weights # 运行模拟退火算法 temperature = INITIAL_TEMPERATURE for iteration in range(NUM_ITERATIONS): # 生成新解 new_weights = current_weights + np.random.normal(0, 0.1, len(mu)) # 计算新解的能量 new_risk, new_returns = calculate_energy(new_weights) # 判断是否接受新解 if new_risk < current_risk or random.uniform(0, 1) < np.exp((current_risk - new_risk) / temperature): current_weights, current_risk, current_returns = new_weights, new_risk, new_returns # 更新最优解 if current_risk < best_risk: best_risk, best_returns = current_risk, current_returns best_weights = current_weights # 降低温度 temperature = INITIAL_TEMPERATURE * np.exp(-iteration / NUM_ITERATIONS * np.log(INITIAL_TEMPERATURE / FINAL_TEMPERATURE)) print(best_weights)注释每一行代码
时间: 2024-02-13 17:00:01 浏览: 26
```python
import numpy as np
import random
# 定义能量函数
def calculate_energy(weights):
# 计算投资组合的风险和收益
# 根据权重计算投资组合的收益
returns = np.dot(mu, weights)
# 根据权重计算投资组合的风险(标准差)
risk = np.sqrt(np.dot(weights.T, np.dot(S, weights)))
return risk, returns
# 模拟退火算法参数
INITIAL_TEMPERATURE = 100.0
FINAL_TEMPERATURE = 0.1
NUM_ITERATIONS = 1000
# 初始化权重
current_weights = np.ones(len(mu)) / len(mu)
# 初始化能量和最优解
current_risk, current_returns = calculate_energy(current_weights)
best_risk, best_returns = current_risk, current_returns
best_weights = current_weights
# 运行模拟退火算法
temperature = INITIAL_TEMPERATURE
for iteration in range(NUM_ITERATIONS):
# 生成新解
new_weights = current_weights + np.random.normal(0, 0.1, len(mu))
# 计算新解的能量
new_risk, new_returns = calculate_energy(new_weights)
# 判断是否接受新解
if new_risk < current_risk or random.uniform(0, 1) < np.exp((current_risk - new_risk) / temperature):
current_weights, current_risk, current_returns = new_weights, new_risk, new_returns
# 更新最优解
if current_risk < best_risk:
best_risk, best_returns = current_risk, current_returns
best_weights = current_weights
# 降低温度
temperature = INITIAL_TEMPERATURE * np.exp(-iteration / NUM_ITERATIONS * np.log(INITIAL_TEMPERATURE / FINAL_TEMPERATURE))
# 输出最优解
print(best_weights)
```
这段代码实现了模拟退火算法来优化一个投资组合的权重分配,使得投资组合的风险最小,同时收益最大。算法的具体步骤如下:
1. 定义能量函数 `calculate_energy`,用于计算投资组合的风险和收益。其中,`mu` 是一个向量,表示不同资产的预期收益率;`S` 是一个协方差矩阵,表示不同资产之间的相关性;`weights` 是一个向量,表示不同资产的权重分配。
2. 初始化模拟退火算法的参数,包括初始温度、最终温度和迭代次数。
3. 初始化投资组合的权重和能量,即初始解。
4. 运行模拟退火算法的迭代过程。每次迭代,生成一个新解,计算新解的能量,并根据 Metropolis 准则判断是否接受新解。
5. 如果接受新解,则更新当前解,并判断是否更新最优解。
6. 降低温度,使得模拟退火算法在后续迭代中更倾向于接受较优解。
7. 最终输出最优解。
这段代码的输出为最优的权重分配。
相关推荐
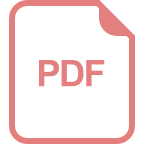
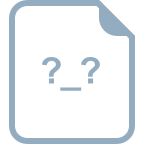
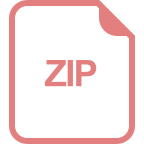














