用c语言输入字符串s1和s2,判断s1中是否包含有s2,如果没有,输出-1,如果有,输出s2在s1中每次出现时的开始位置,位置从0开始。
时间: 2024-09-30 15:02:13 浏览: 70
在 C 语言中,你可以通过遍历字符串 s1 来查找字符串 s2 是否存在于其中。这里是一个简单的函数示例,它实现了这个功能:
```c
#include <stdio.h>
#include <string.h>
void find_substring(char *s1, char *s2) {
int len_s1 = strlen(s1);
int len_s2 = strlen(s2);
if (len_s2 > len_s1) {
printf("-1\n"); // 如果 s2 长度大于 s1,说明 s2 不可能在 s1 中
return;
}
for (int i = 0; i <= len_s1 - len_s2; i++) {
int match_count = 0;
// 检查 s2 是否连续出现在 s1 的子串中
for (int j = 0; j < len_s2; j++) {
if (s1[i + j] != s2[j]) {
break;
}
match_count++;
}
// 如果找到完全匹配,输出起始位置并继续寻找下一个子串
if (match_count == len_s2) {
printf("%d ", i);
}
}
}
int main() {
char s1[100], s2[100];
printf("请输入字符串 s1: ");
fgets(s1, sizeof(s1), stdin); // 注意处理换行符
s1[strlen(s1)-1] = '\0'; // 去除fgets获取的最后一字符可能是换行符
printf("请输入字符串 s2: ");
fgets(s2, sizeof(s2), stdin);
s2[strlen(s2)-1] = '\0';
find_substring(s1, s2);
return 0;
}
```
当你运行此程序时,它会提示用户输入两个字符串,然后检查 `s1` 是否包含 `s2`。如果存在,它将输出 `s2` 在 `s1` 中的每个完整匹配位置。
阅读全文
相关推荐
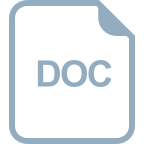
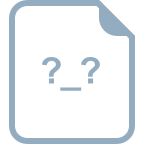
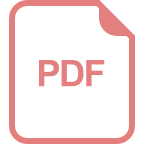















