spring boot mybatis puls 查找一个表的所有数据,并带出他对应子类的总数
时间: 2023-12-06 14:45:20 浏览: 56
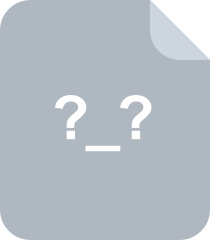
Springboot集成MyBatis实现查询表操作
假设你的表名为 `parent_table`,有一个子类表 `child_table`,并且 `child_table` 中有一个外键 `parent_id` 指向 `parent_table` 的主键 `id`,你可以先定义两个实体类 `Parent` 和 `Child`,然后使用 MyBatis-Plus 提供的方法进行查询。
1. 定义实体类
Parent.java
```java
@Data
public class Parent {
private Long id;
private String name;
private List<Child> children;
}
```
Child.java
```java
@Data
public class Child {
private Long id;
private String name;
private Long parentId;
}
```
2. 定义 Mapper 接口
ParentMapper.java
```java
@Mapper
public interface ParentMapper extends BaseMapper<Parent> {
List<Parent> selectParentsWithChildren();
}
```
3. 实现 Mapper 接口
ParentMapper.xml
```xml
<mapper namespace="com.example.mapper.ParentMapper">
<resultMap id="parentResultMap" type="com.example.entity.Parent">
<id column="id" property="id"/>
<result column="name" property="name"/>
<collection property="children" ofType="com.example.entity.Child">
<id column="id" property="id"/>
<result column="name" property="name"/>
<result column="parent_id" property="parentId"/>
</collection>
</resultMap>
<select id="selectParentsWithChildren" resultMap="parentResultMap">
SELECT p.*, COUNT(c.id) AS child_count
FROM parent_table p
LEFT JOIN child_table c ON p.id = c.parent_id
GROUP BY p.id;
</select>
</mapper>
```
4. 使用 Mapper 进行查询
```java
@Service
public class ParentService {
@Autowired
private ParentMapper parentMapper;
public List<Parent> getParentsWithChildren() {
return parentMapper.selectParentsWithChildren();
}
}
```
这样,你就可以查询出所有 `parent_table` 的数据,并且每个 `Parent` 对象中都带有其对应的子类总数。
阅读全文
相关推荐
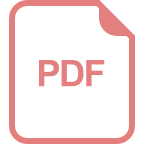
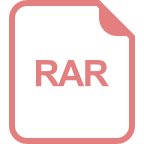
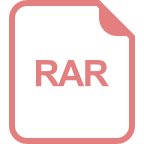
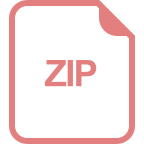
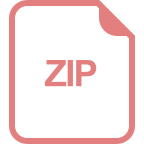
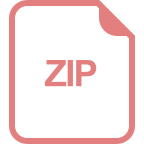