java 根据网络地址下载文件
时间: 2023-11-17 09:47:57 浏览: 83
你可以使用Java中的URLConnection类和InputStream类来实现根据网络地址下载文件的功能。
以下是一个基本的示例代码:
```java
import java.io.*;
import java.net.*;
public class Downloader {
public static void downloadFile(String fileURL, String saveDir) throws IOException {
URL url = new URL(fileURL);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
int responseCode = httpConn.getResponseCode();
// 检查 HTTP 响应是否成功
if (responseCode == HttpURLConnection.HTTP_OK) {
// 获取文件名
String fileName = "";
String disposition = httpConn.getHeaderField("Content-Disposition");
String contentType = httpConn.getContentType();
int contentLength = httpConn.getContentLength();
if (disposition != null) {
// 从 Content-Disposition 中获取文件名
int index = disposition.indexOf("filename=");
if (index > 0) {
fileName = disposition.substring(index + 10, disposition.length() - 1);
}
} else {
// 从 URL 中获取文件名
fileName = fileURL.substring(fileURL.lastIndexOf("/") + 1, fileURL.length());
}
// 输出文件信息
System.out.println("Content-Type = " + contentType);
System.out.println("Content-Disposition = " + disposition);
System.out.println("Content-Length = " + contentLength);
System.out.println("fileName = " + fileName);
// 打开网络输入流
InputStream inputStream = httpConn.getInputStream();
// 打开本地文件输出流
String saveFilePath = saveDir + File.separator + fileName;
FileOutputStream outputStream = new FileOutputStream(saveFilePath);
// 从网络输入流读取数据,写入本地文件输出流
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
// 关闭流
outputStream.close();
inputStream.close();
System.out.println("文件下载成功");
} else {
System.out.println("文件下载失败,HTTP 响应状态码为 " + responseCode);
}
httpConn.disconnect();
}
public static void main(String[] args) {
String fileURL = "http://example.com/file.zip";
String saveDir = "C:/Downloads";
try {
downloadFile(fileURL, saveDir);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,`downloadFile`方法接收文件的URL和保存目录作为参数,并使用`HttpURLConnection`打开连接。我们检查响应代码以确保连接成功,然后解析响应头中的文件名和其他信息。最后,我们使用`InputStream`从网络输入流读取数据,并使用`FileOutputStream`将数据写入本地文件输出流。最后,我们关闭流并断开连接。
阅读全文
相关推荐
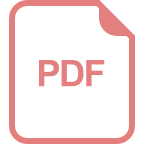
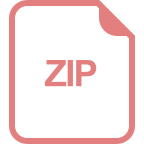
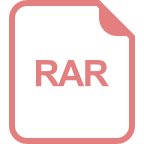
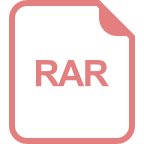
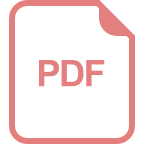
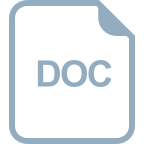
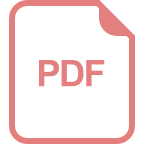
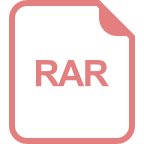
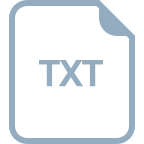
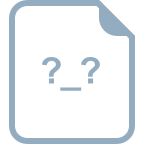
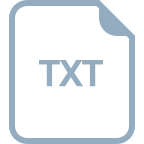
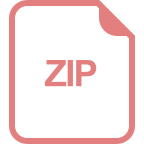