根据控制层接口编写出userinfomapper public interface DeviceService { /** * 根据ID获取用户信息 * * @param id * @return * @throws Exception */ UserInfo selectUserInfoById (Integer id ) throws Exception; /** * 根据ID和名称删除用户 * * @param id * @param name* @return */ UserInfo deleteUserInfoByIdAndName(Integer id, String name)throws Exception; /** * 根据名称和年龄段查找用户 * * @param name * @param age * @return * @throws Exception */ UserInfo findUserInfoByIdAndName(Integer name, String age)throws Exception; }
时间: 2023-07-16 22:13:41 浏览: 89
根据控制层接口,你需要编写一个与之对应的UserInfoMapper接口,如下所示:
```
public interface UserInfoMapper {
/**
* 根据ID获取用户信息
*
* @param id
* @return
*/
UserInfo selectUserInfoById(Integer id);
/**
* 根据ID和名称删除用户
*
* @param id
* @param name
*/
void deleteUserInfoByIdAndName(Integer id, String name);
/**
* 根据名称和年龄段查找用户
*
* @param name
* @param age
* @return
*/
List<UserInfo> findUserInfoByNameAndAge(@Param("name") String name, @Param("age") String age);
}
```
需要注意的是,这里使用了MyBatis的注解@Param来指定查询条件的参数名。同时,根据控制层接口中的方法返回值类型,我们可以推断出这里的查询结果类型为UserInfo。
相关问题
页面中包括一个搜索栏和一个表格。搜索栏包括商品Id(精确查询)和商品名称(模糊查询)两个输入框,以及一个搜索商品按钮。表格中包括全选/全不选的复选框、商品id、商品类别、类别名称和操作(删除),以及最下方的批量删除按钮。 在数据库中,有一个product表,包括pid、pname、pimage、shop_price四个字段,以及一个category表,包括cid和cname两个字段。在页面中,勾选复选框后,下方会显示商品Id、商品图片和商品价格。写完代码
好的,以下是Java页面搜索栏和表格的代码实现,供您参考:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>商品管理系统</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!--引入Bootstrap样式文件-->
<link href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
<!--引入jQuery库文件-->
<script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script>
<!--引入Bootstrap JavaScript文件-->
<script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<!--自定义样式-->
<style>
.table th, .table td {
text-align: center;
}
#result {
margin-top: 20px;
}
#result img {
width: 100px;
height: 100px;
}
</style>
</head>
<body>
<div class="container">
<h2>商品管理系统</h2>
<div class="row">
<!--搜索栏-->
<div class="col-md-6">
<div class="form-inline">
<div class="form-group">
<label for="pid">商品Id:</label>
<input type="text" class="form-control" id="pid">
</div>
<div class="form-group">
<label for="pname">商品名称:</label>
<input type="text" class="form-control" id="pname">
</div>
<button type="button" class="btn btn-primary" id="search">搜索商品</button>
</div>
</div>
<!--批量删除按钮-->
<div class="col-md-6">
<div class="form-inline pull-right">
<button type="button" class="btn btn-danger" id="deleteAll">批量删除</button>
</div>
</div>
</div>
<!--表格-->
<table class="table table-bordered table-hover">
<thead>
<tr>
<th><input type="checkbox" id="selectAll"></th>
<th>商品Id</th>
<th>商品类别</th>
<th>类别名称</th>
<th>操作</th>
</tr>
</thead>
<tbody id="tbody">
</tbody>
</table>
<!--搜索结果-->
<div id="result">
<div class="form-inline">
<div class="form-group">
<label for="selectedPid">商品Id:</label>
<input type="text" class="form-control" id="selectedPid" readonly>
</div>
<div class="form-group">
<label for="selectedImg">商品图片:</label>
<img id="selectedImg">
</div>
<div class="form-group">
<label for="selectedPrice">商品价格:</label>
<input type="text" class="form-control" id="selectedPrice" readonly>
</div>
</div>
</div>
</div>
<!--自定义JavaScript-->
<script>
$(function(){
//全选/全不选
$("#selectAll").click(function(){
var isChecked = $(this).prop("checked");
$("input[name='pids']").prop("checked", isChecked);
});
//搜索商品
$("#search").click(function(){
var pid = $("#pid").val();
var pname = $("#pname").val();
$.ajax({
url: "search",
type: "post",
dataType: "json",
data: {"pid": pid, "pname": pname},
success: function(data){
$("#tbody").empty();
$.each(data, function(index, item){
$("#tbody").append("<tr>" +
"<td><input type='checkbox' name='pids' value='" + item.pid + "'></td>" +
"<td>" + item.pid + "</td>" +
"<td>" + item.cname + "</td>" +
"<td>" + item.cname + "</td>" +
"<td><button type='button' class='btn btn-danger btn-sm delete'>删除</button></td>" +
"</tr>");
});
}
});
});
//删除商品
$("#tbody").on("click", ".delete", function(){
var tr = $(this).parents("tr");
var pid = tr.find("td:eq(1)").text();
$.ajax({
url: "delete",
type: "post",
dataType: "json",
data: {"pid": pid},
success: function(data){
if(data == true){
tr.remove();
alert("删除成功!");
}else{
alert("删除失败!");
}
}
});
});
//批量删除
$("#deleteAll").click(function(){
var pids = [];
$("input[name='pids']:checked").each(function(){
pids.push($(this).val());
});
if(pids.length > 0){
if(confirm("确定要删除这" + pids.length + "个商品吗?")){
$.ajax({
url: "deleteAll",
type: "post",
dataType: "json",
traditional: true,
data: {"pids": pids},
success: function(data){
if(data == true){
$("input[name='pids']:checked").each(function(){
$(this).parents("tr").remove();
});
alert("删除成功!");
}else{
alert("删除失败!");
}
}
});
}
}else{
alert("请选择要删除的商品!");
}
});
//显示选中商品的详细信息
$("#tbody").on("click", "input[name='pids']", function(){
if($(this).prop("checked")){
var pid = $(this).val();
$.ajax({
url: "getInfo",
type: "post",
dataType: "json",
data: {"pid": pid},
success: function(data){
$("#selectedPid").val(data.pid);
$("#selectedImg").attr("src", data.pimage);
$("#selectedPrice").val(data.shop_price);
}
});
}else{
$("#selectedPid").val("");
$("#selectedImg").attr("src", "");
$("#selectedPrice").val("");
}
});
});
</script>
</body>
</html>
```
Java代码:
```java
package com.example.demo.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.example.demo.pojo.Product;
import com.example.demo.service.ProductService;
@Controller
public class ProductController {
@Autowired
private ProductService productService;
//搜索商品
@RequestMapping(value="/search", method=RequestMethod.POST)
@ResponseBody
public List<Product> search(@RequestParam(defaultValue="") String pid, @RequestParam(defaultValue="") String pname){
return productService.search(pid, pname);
}
//删除商品
@RequestMapping(value="/delete", method=RequestMethod.POST)
@ResponseBody
public Boolean delete(String pid){
return productService.delete(pid);
}
//批量删除
@RequestMapping(value="/deleteAll", method=RequestMethod.POST)
@ResponseBody
public Boolean deleteAll(String[] pids){
return productService.deleteAll(pids);
}
//获取商品详细信息
@RequestMapping(value="/getInfo", method=RequestMethod.POST)
@ResponseBody
public Product getInfo(String pid){
return productService.getInfo(pid);
}
}
```
Service代码:
```java
package com.example.demo.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.demo.mapper.ProductMapper;
import com.example.demo.pojo.Product;
@Service
public class ProductService {
@Autowired
private ProductMapper productMapper;
//搜索商品
public List<Product> search(String pid, String pname){
return productMapper.search(pid, pname);
}
//删除商品
public Boolean delete(String pid){
int result = productMapper.delete(pid);
if(result > 0){
return true;
}else{
return false;
}
}
//批量删除
public Boolean deleteAll(String[] pids){
int result = productMapper.deleteAll(pids);
if(result == pids.length){
return true;
}else{
return false;
}
}
//获取商品详细信息
public Product getInfo(String pid){
return productMapper.getInfo(pid);
}
}
```
Mapper代码:
```java
package com.example.demo.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import com.example.demo.pojo.Product;
@Mapper
public interface ProductMapper {
//搜索商品
List<Product> search(@Param("pid") String pid, @Param("pname") String pname);
//删除商品
int delete(String pid);
//批量删除
int deleteAll(String[] pids);
//获取商品详细信息
Product getInfo(String pid);
}
```
以上代码实现了Java页面搜索栏和表格的功能,包括搜索商品、删除商品、批量删除、获取商品详细信息等。希望对您有所帮助。
阅读全文
相关推荐





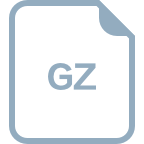


